Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial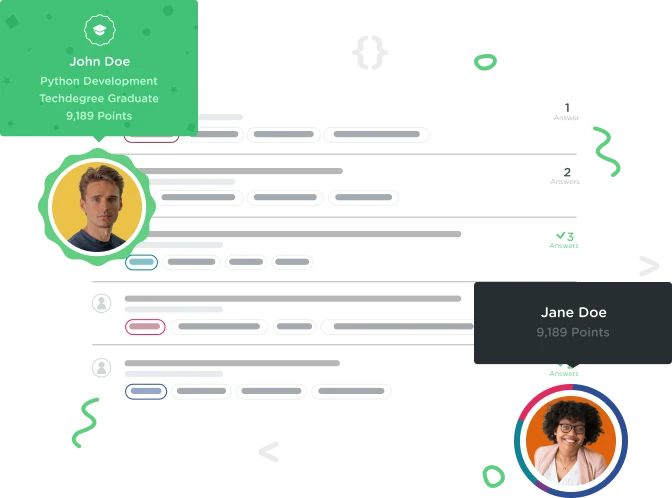

Thomas Katalenas
11,033 Pointsmessy_list = [5,2,1,3,4,7,8,0,9,-1] silly_list = messy_list[12:0:-3]
messy_list = [5,2,1,3,4,7,8,0,9,-1] so create a variable named silly_list that is suppossed to create the same list in reverse and take every three out of that list
silly_list = messy_list[12 :0:-3] returns [-1, 8, 3]
why isn't this right in the console
1 Answer

Jeff Wilton
16,646 PointsClassic case on how to overthink the problem (happens to me all the time) :) But it brings up an important lesson on Python slicing and indexing.
First the solution:
As you probably know, you can copy an entire list by doing this:
list_2 = list_1[:]
We didn't have to specify the start and stop indexes since we want the whole list. Same thing is true in reverse:
silly_list = messy_list[::-3]
print silly_list
[-1, 8, 3, 5]
We could be done there since you now have the solution, but let me explain some of what is happening automatically there. In the above case [::-3], think of it like this:
[a : b : -3]
a is by default -1
b is by default -(len+1)
Thus the statement could be explicitly stated as follows:
silly_list = messy_list[-1:-11:-3]
print silly_list
[-1, 8, 3, 5]
I'm sure they set this assignment up this way intentionally to help people understand how Python slicing works. Hope this helps!