Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial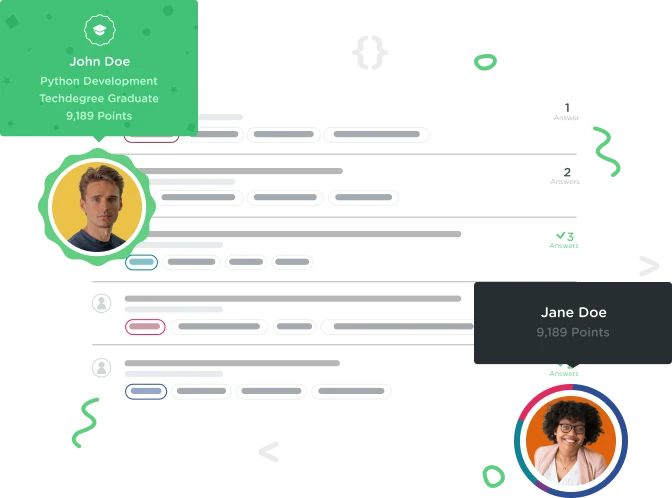

Jose Leonardo
850 PointsPezDispenzer dispenzer = new PezDispenzer(); Why do we use "New"?
Why do we use the parameter New although the class is already clearly defined?
3 Answers
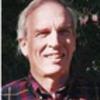
jcorum
71,830 PointsYou use new (not New) to create objects. PezDispenser is a class. If you want PezDispenser objects (instances of the class) you need to create them. Here we create two of them:
PezDispenser pd1 = new PezDispenser();
PezDispenser pd2 = new PezDispenser();
Here I'm assuming the PezDispenser constructor doesn't expect any parameters.
Take another example. If you have a Student class, you don't have any Student objects until you create them using new:
Student jose = new Student("Jose");
Student bill = new Student("Bill");
Student maria = new Student("Maria");
Here I'm assuming that the Student constructor requires a name.
Now there are three Student objects, or 3 instances of the Student class.
Remember, Java is case-sensitive, so new and New are different.
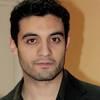
Carlos Federico Puebla Larregle
21,074 PointsThe keyword "new" is not a parameter is an operator and you have to "postfix" to the "new" operator a call to the constructor in this case "PezDispenzer();". The new operator returns a reference to the object it created. This reference is usually assigned to a variable of the appropriate type, in this case the variable "dispenzer" of type "PezDispenzer".
I hope that helps a little bit.

Jon Sultana
6,916 PointsThe new keyword I am assuming is used for Dynamic memory allocation. I am a former C++ student and we would use the new keyword whenever we wanted to dynamically allocate memory in the heap. Once the program ends or the object goes out of scope the memory is released back to the free store. So what it seems like you are doing is creating two new PezDispenser objects dynamically by calling the default object constructor that you do not see because the compiler takes care of it for you"PezDispenser()".