Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial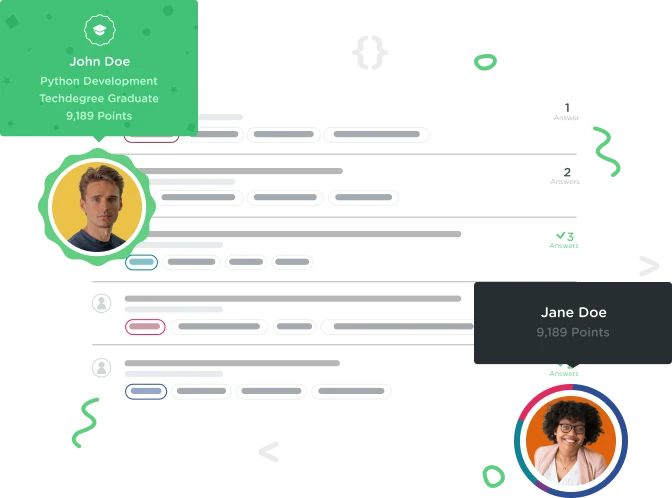

Tyrone Kappenhagen
504 PointsPutting the "Fun" Back in "Function" Understanding my solution
I successfully solved the both parts of the challenge; however, I would love a better explanation of what exactly is going on in #Part 1 of my code. Specifically, why did I need to define total and set it =0?
Code:
Part 1
def add_list(lst): total = 0 for item in lst: total += item return total
Part 2
def summarize(lst): return("The sum of {} is {}.".format(lst, add_list(lst)))
3 Answers
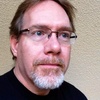
Chris Freeman
Treehouse Moderator 68,423 PointsLooking the values each time through the loop, the addition line can be thought of as:
# increment old_total to make new_total
new_total = old_total + item
# before start of next loop:
# save the previous value as old_value
old_total = new_total
The loop would unroll like this:
total_1 = total_initial + firs_item
total_2 = total_1 + second_item
total_3 = total_2 + third_item
# which becomes, in the case of [1, 2, 3]
total = 0 + 1
total = 1 + 2
total = 3 + 3
# total now equals 6
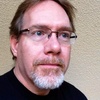
Chris Freeman
Treehouse Moderator 68,423 PointsIn the code:
def add_list(lst):
total = 0
for item in lst:
total += item
return total
# the line ...
total += item
# is equivalent to
total = total + 1
When this line is evaluated on the first pass through the for
loop
the right-hand side is evaluated be before the left. Also at this point
"total" doesn't yet have a value, so total + 1
can't be done. This will throw an undefined variable
error. By setting "total" to 0 before the loop, the initial value is set for
the first pass through the loop.
Does that make sense?

Tyrone Kappenhagen
504 PointsThat actually does make sense and I completely overlooked the fact that I was looping the code (i.e. "for.."). So each time 'item' loops through it's the same as:
total = 0 +1
total = 0 +2
total = 0 + 3
am I correct in thinking this?
Thus, the 2nd half of the code returns
def summarize(lst):
return "The sum of {} is {}.".format(lst, add_list(lst))
#OR
return "The sum of [1, 2, 3] is 6"?
[MOD: added ```python formatting. -cf]

Tyrone Kappenhagen
504 PointsMakes perfect sense! Thank you again.