Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial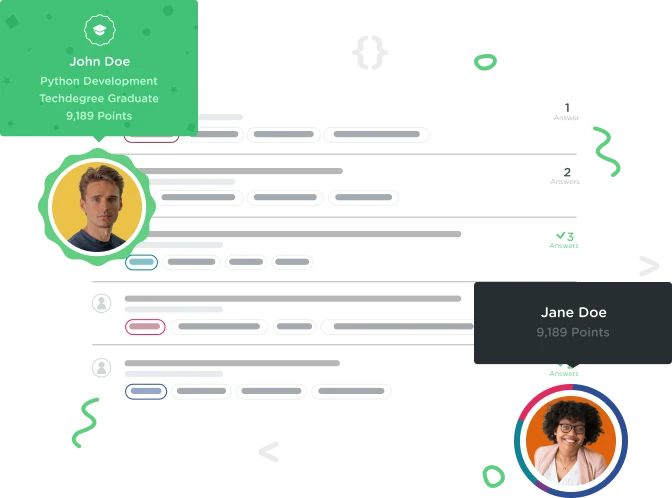

Sam Morpeth
8,756 PointsStudent record search challenge - Extra credit challenge
So I'm struggling with one of the extra credit challenges from the Javascript course. The idea is to add an additional message when the student is not in the array of objects "students", rather than simply doing nothing.
I spent a while trying to figure out how I can use if/else/else if statements, but when they're nested I find it very confusing.
My initial idea was to test whether or not the student was in the list by using the indexOf function and comparing it to see if it was more than -1, and then have the rest of the program run if that was true. However, it seems to print the message even if the student is in the list of students.
Additionally after much frustration and iterations I can't seem to remember how I tried to implement that and have ended up with the code below in which I've tacked on the code I'm trying to make check if the student is there or not. I think both attempts ended up with the same result.
I have a feeling that somehow I have accidentally made the initial condition of checking whether they are in the list of students always true somehow, but I don't know why or how I've done that.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Please enter the name of a student e.g. 'Judy' to find information about that student. When you're finished type 'quit' to exit");
if (search === null || search.toLowerCase() === "quit") {
break;
} else {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message = getStudentReport( student );
print(message);
} else {
if (students.indexOf(search) === -1) {
print(search + " ain't here.");
}
}
}
}
}
1 Answer

Jon Boettcher
7,343 PointsThe first thing that should help in making your nested statements easier to understand is that your first else
clause isn't needed in this case:
if (search === null || search.toLowerCase() === "quit") {
break;
} else {
The first condition being tested will break out of the loop completely if search
is equal to null
or "quit", so therefore any other values will cause the loop to continue. In my opinion it's cleaner to remove the else
completely to avoid any unnecessary nesting. As in:
if (search === null || search.toLowerCase() === "quit") {
break;
}
for (var i = 0; i < students.length; i += 1) {
...
For your searching strategy, using the indexOf()
function is going to cause some problems because of the data structure being used for students
. students
is an array of objects and those objects in turn have both strings and numbers. By using the call of students.indexOf(search)
, you are trying to search for a string element (search
) in the students
array. Since all of the elements in the array are objects and not strings, the value is always going to come up as -1.
You may also want to ask yourself if that search is needed at all. You're already checking if the student exists:
if ( student.name === search )
If that condition is true
, then the student exists and if it's false
, then the for
loop should continue on through the rest of the student records and check if any of those are a match. You won't know if the student exists or not until each record is checked.
Hopefully that makes sense and helps a bit.