Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial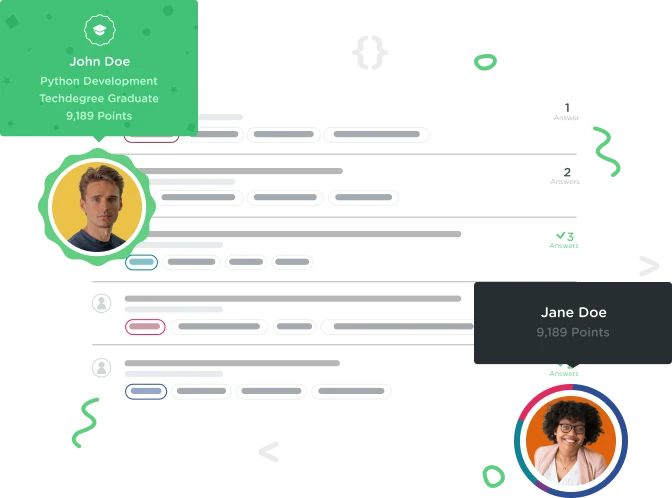
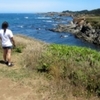
Nancy Melucci
Courses Plus Student 35,157 PointsThrowing an exception in a GUI interface from the setter (Java)
I have been assigned to create a GUI interface linked to a Rectangle class. The interface takes input from the user and returns the dimensions (area, perimeter). No image. I have been asked as part of this to have the setter that takes height and width throw an exception that appears in a JOptionPane if the input is out of range (0 or negative.) There is an exception in the main class for non-numeric input (that works) but I cannot make the exception throw AND make the dialog box show it. I put in negative numbers and a try-catch that compiles but it just barrels on thru making a negative area rectangle. How can I make it throw the error and show it in a GUI message box. Code for main class (Swing) and Rectangle below. The basic rectangle thing works.
import javax.swing.JOptionPane;
public class Assign8 extends javax.swing.JDialog {
/**
* Creates new form Assign8
*/
public Assign8(java.awt.Frame parent, boolean modal) {
super(parent, modal);
initComponents();
}
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 400, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGap(0, 300, Short.MAX_VALUE)
);
pack();
}// </editor-fold>
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
String firstNumber
= JOptionPane.showInputDialog("Enter the height");
String secondNumber
= JOptionPane.showInputDialog("Enter the width");
try {
double width = Double.parseDouble(firstNumber);
double height = Double.parseDouble(secondNumber);
Rectangle guiRect = new Rectangle(width, height);
JOptionPane.showMessageDialog(null, guiRect.toString(), "Your new rectangle", JOptionPane.PLAIN_MESSAGE);
} catch (IllegalArgumentException iae) {
JOptionPane.showMessageDialog(null, "Please provide numeric input only", "Invalid input", JOptionPane.PLAIN_MESSAGE);
}
}
}
import java.text.DecimalFormat;
//class Rectangle
public class Rectangle {
double width;
double height;
//constructor
public Rectangle() {
width = 1;
height = 1;
}
//constructor
public Rectangle(double w, double hi) {
this.width = w;
this.height = hi;
}
//setters
public void setWidth(double w) {
try {
width = w;
} catch (IllegalArgumentException iae) {
if (width <= 0) {
throw new IllegalArgumentException("Invalid value: " + width);
}
}
}
public void setHeight(double hi) {
{
try {
height = hi;
} catch (IllegalArgumentException iae) {
if (height <= 0) {
throw new IllegalArgumentException(
"Invalid value: " + height);
}
}
}
}
//getters
public double getWidth() {
return width;
}
public double getHeight() {
return height;
}
//the methods of the class
public double getArea() {
return width * height;
}
public double getPerimeter() {
return (2 * height) + (2 * width);
}
//to String
@Override
public String toString() {
DecimalFormat format = new DecimalFormat("#.00");
return "The height of the rectangle is " + this.getHeight() + " \n The width of the rectangle is " + this.getWidth() + "\n"
+ "The perimeter of the rectangle is " + format.format(this.getPerimeter()) + "\n"
+ "The area of the Rectangle is " + format.format(this.getArea()) + "\n";
}
}
3 Answers
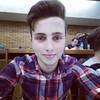
Rares Conea
Courses Plus Student 15,000 PointsHi, Your setters are not defined correctly. First of all, if you pass a negative value to one of the setters what exception would you expect to occur? The catch block is run only if an exception is thrown and your code is not defined to behave this way. The code for the setters should be: https://imgur.com/a/mpFh8 . The other setter should look the same.
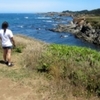
Nancy Melucci
Courses Plus Student 35,157 PointsThe setter works better (thank you) but the problem is that it (the exception) still doesn't send a message to the main part of the assignment - the part that makes the GUI - I am supposed to show an error message in a dialog box that tells the user that the input is invalid. It's still making a rectangle with a negative area.
I can't work on this more today because of the holiday. I will check for more guidance tomorrow. I am trying to cross the foggy bridge and I am thankful for the community here. A Happy holiday where applicable!
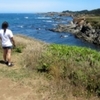
Nancy Melucci
Courses Plus Student 35,157 PointsMade one stride forward...I have to explicitly call the setter in my swing app/main method.
I have to add code like this:
guiRect.setWidth(width);
guiRect.setHeight(height);
After I create the rectangle:
Rectangle guiRect = new Rectangle(width, height);
Then it throws the exception. I still have some problems left to solve, but I have 6 days. Will keep working on it (obvi)
thanks.
Rares Conea
Courses Plus Student 15,000 PointsRares Conea
Courses Plus Student 15,000 PointsThe if statement should be hi <= 0.