Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial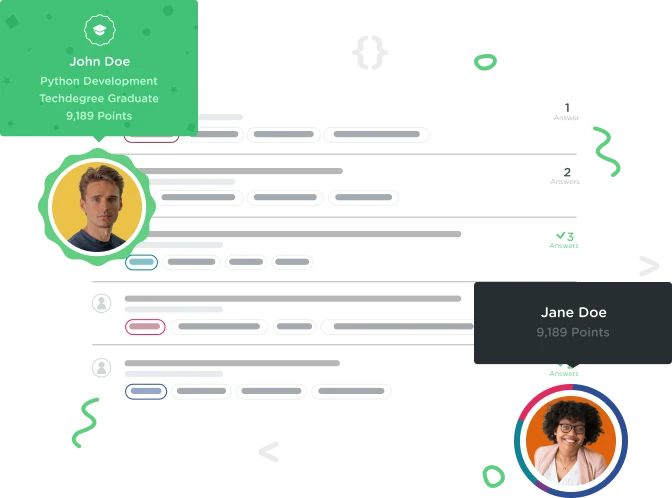

David Aguirre
8,170 Points=== true ? false : true;
can somebody refresh me of what this syntax actually does? === true ? false : true;
Also I tried to iterate with a normal forloop
What (let player of this.players) actually do? More precisely it's just saying iterate the amount of arrays of this.players?

anmo20
6,470 PointsI don't understand why they are asking to iterate at all. It's only 2 values. I figured an IF statement would work just fine.
I would just use an IF statement like this:
switchPlayers () {
if (this.players[0].active === true) {
this.players[0].active = false;
this.players[1].active = true;
} else {
this.players[0].active = true;
this.players[1].active = false;
}
}
2 Answers
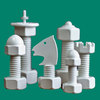
Steven Parker
230,274 PointsAndrew's already explained the ternary operator, but if you want more details, here's a link to the MDN page on Conditional Operator.
But this particular one is inverting a boolean, which can be done much more concisely:
// these three expressions are equivalent:
someval === true ? false : true // inverts a boolean
soveval ? false : true // but you never need to compare to "true"
!someval // and the "not" operator is designed for this
And "for (let player of this.players)
" is a loop that will go through the iterable in this.players assigning "player" with the contents of one of the elements in each iteration. For more details on that, here's the MDN page on "for...of".
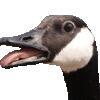
wildgoosestudent
11,274 PointsThese were the two options I cam up with after a bit of research. Both work, but I think the ternary (option 2) is a bit more readable.
I call switchPlayers()
at the end of the playToken()
method and then added in some console.log()
s so that I can see what it actually happening.
switchPlayers() {
for (let player of this.players) {
console.log(player);
player.active = !player.active;
player.active = player.active? false : true;
console.log(player);
}
}
Andrew Phythian
19,747 PointsAndrew Phythian
19,747 PointsThe syntax you have posted is called a Ternary Operator. For more information about it look at the Mozilla docs linked on the next line. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Conditional_Operator
condition ? exprT : exprF
It tests a condition then returns two different outcomes whether the condition is true or false. For example let's say I have a variable x and want to return something different depending on the value of x.
if (x == 20) ? 'Yes' : 'No'
When x holds the value 20, the operator returns the string 'Yes', otherwise it returns 'No.
Ternary operators are a shorter and more efficient way of running a traditional 'if' statement.