Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial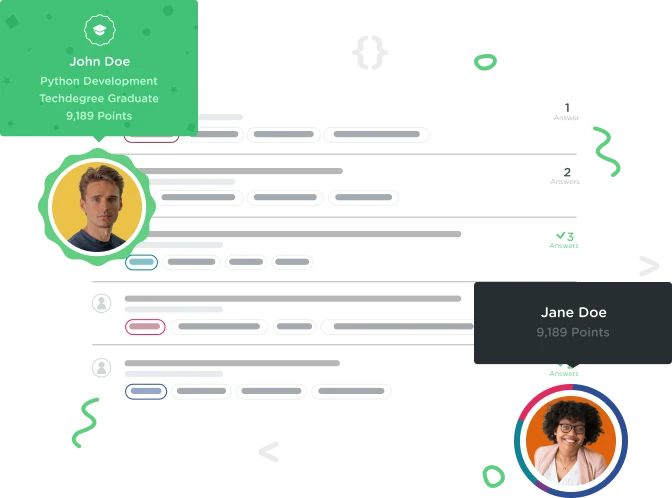

Sandra Jenniffer Dheming Lemus
Courses Plus Student 939 PointsvideoGame.Characters = {"charactername1", "charactername2"}; not working in controller initialization
I keep getting error when initializing the array from the model, any suggestions?
using System.Web.Mvc;
using Treehouse.Models;
namespace Treehouse.Controllers
{
public class VideoGamesController : Controller
{
public ActionResult Detail()
{
var videoGame = new VideoGame();
videoGame.Title = "Super Mario 64";
videoGame.Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.";
videoGame.Characters = {
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi" };
return View(videoGame);
}
}
}
@ model VideoGamesController.Models.VideoGames
@{
ViewBag.PageTitle = "Video Game Detail";
}
<h1>@Model.Title</h1>
<h5>Description:</h5>
<div>@Model.Description</div>
<h5>Characters:</h5>
<div>
<ul>
@foreach (var character in Model.Characters)
{
<li>@character</li>
}
</ul>
</div>
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
1 Answer

James Churchill
Treehouse TeacherSandra,
Sorry for the slow response! Hopefully you were able to get past this issue. But just in case you haven't...
Before you can initialize an array in C#, you might need to construct it using the new
keyword followed by the type of the items that the array will contain. Here's an example of what that looks like:
var characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
};
If you replace the var
keyword with the specific array type when declaring my variable, you can use this shorter syntax:
string[] characters =
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
};
If you have a class that defines an array property, like the VideoGame class in the code challenge that you're trying to solve, then you need to do this when initializing the property with a value:
var videoGame = new VideoGame();
videoGame.Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
};
Or this works too (notice that the string
keyword has been removed):
var videoGame = new VideoGame();
videoGame.Characters = new []
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
};
I hope this helps.
Thanks ~James