Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial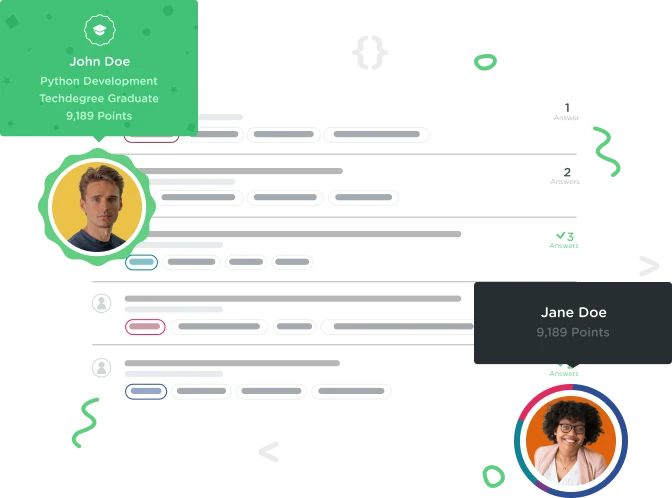
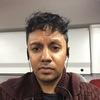
Akash Sharma
Full Stack JavaScript Techdegree Student 14,147 Points2 column table attempt
app.js file
app.get('/sandbox', (req,res) => {
res.render('sandbox',{firstName, lastName});
});
const firstName = ["Will","Leo","James","Paula","Larry"];
const lastName = ["Smith","Di Caprio","Franco","Patton","David"];
sandbox.pug
doctype html
html(lang="en")
head
title Friends Table
body
table
tr
th
h3 First Name
th
h3 Last Name
tr
td
each val in firstName
p= val
td
each val in lastName
p= val
Wanted to do a nested object but could not find patience so iterate over.
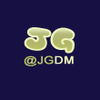
Jonathan Grieve
Treehouse Moderator 91,253 PointsI followed your example adrian, but to do it, I had to use the interpolation syntax. As below.
table
tr
th First Name
th Last Name
each name in names
tr
td #{name.split( ' ' )[0]}
td #{name.split( ' ' )[1]}
2 Answers

Rob Inman
4,516 PointsI did mine a little bit differently:
app.js
const sandboxNames = [{First: 'Paul', Last: 'Jones'},
{First: 'David', Last: 'Smith'},
{First: 'Jason', Last: 'Camp'},
{First: 'Bella'},
{Last: 'Jones'}];
sandbox.pug
doctype html
html(lang="en")
head
title Sandbox Example
body
if sandboxNames
table(style="width:50%")
col(width="100")
col(width="100")
th First
th Last
each name in sandboxNames
tr
if name.First
th= name.First
else
th First Name Not Given
if name.Last
th= name.Last
else
th Last Name Not Given

Marko Delgadillo
4,524 Pointsapp.js
const names = [
{ first: null, last: "Einstein" },
{ first: "Marko", last: "Delgadillo" },
{ first: "Breadna", last: "Pancakes" },
{ first: "Karen", last: "Dahmer" }
];
card.pug
if names
table
tr
th First
th Last
each name in names
tr
if name.first
td= name.first
else
td= "N/A"
if name.last
td= name.last
else
td= "N/A"
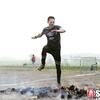
Johnny Austen
Front End Web Development Techdegree Graduate 35,494 PointsI used a multi-dimensional array:
app.js
people = [ ["Johnny", "Bozzman"], ["Tommy", "Dozzman"], ["Rommy", "Szlozshman"] ]
app.get('/sandbox', (req, res) => { res.render('sandbox', {prompt: "Here are some peoplez", people}) })
sandbox.pug
table(style='width:100%', border='1') tr th First th Last each person in people tr th= person[0] th= person[1]
Adrian Orta
30,490 PointsAdrian Orta
30,490 PointsMore than likely users will enter their first and last name as one so: