Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial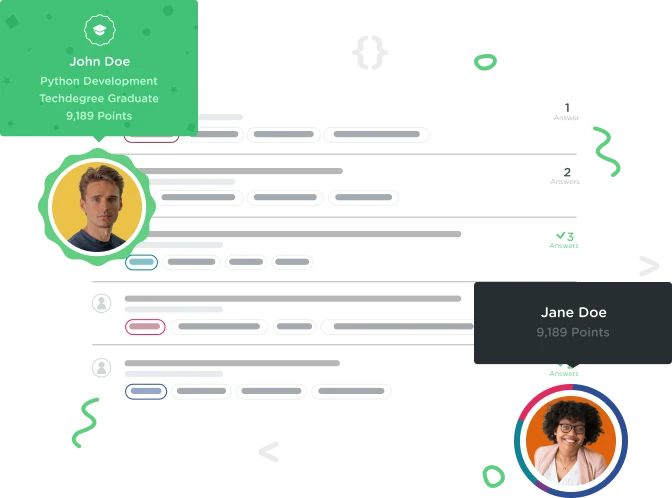

Vijayalaxmi vastrad
2,789 Points2 I have two different div under one section, I want them to fadeIn and fadeaOut randomly within an infinite loop.
//html <section class="main-bg"> <div class="content1"> <h1>viju</h1> <button>submit</button> </div> <div class="content2"> <h1>vagi</h1> <button>Click Me</button> </div> </section> //js $(document).ready(function () { $('content2').hide(); setInterval(function () { $('.content1').delay(1000).fadeIn(2000).fadeOut(2000, function () { $('.content2').fadeIn(4000); }) }, 4000);
});
4 Answers
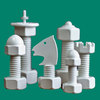
Steven Parker
230,274 PointsYou're getting pretty close. A few hints:
- the period is missing from the "
.content
" selector used with "hide" - "content2" fades in but doesn't fade out
- since "content1" starts visible, you might want to fade it out first
Making those changes:
$(".content2").hide();
setInterval(function () {
$(".content1").delay(1000).fadeOut(2000).fadeIn(2000, function () {
$(".content2").fadeIn(4000).fadeOut(2000);
});
}, 4000);
The only thing left is the "random" aspect you mentioned, though this might already appear to be random to the average observer as it is.
If it's necessary to be more random, the callbacks could create random values for the time settings

Vijayalaxmi vastrad
2,789 PointsI tried above code but its not coming. both contents are showing at time. i want it like first content1 need to appear stay for some time and fadeOut and content2 appear stay for some time and fadeout than again content1 so on... but content1 appearing twice before content2 and than content2 appears and both appears. may be its adjustment of intervals that i am not getting

Vijayalaxmi vastrad
2,789 PointsPerfect i got it exactly how i want, but content1 is appearing too too late after loading the page than sequence is perfect.
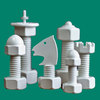
Steven Parker
230,274 PointsThe setInterval code doesn't run until after the first interval elapses. Just perform a similar sequence once outside of the setInterval also:
// after the "setInterval" call:
$(".content1").delay(3000).fadeOut(2000, function() {
$(".content2").fadeIn(2000).delay(1000).fadeOut(2000);
});

Vijayalaxmi vastrad
2,789 Pointssolved! thank you so so much
Steven Parker
230,274 PointsSteven Parker
230,274 PointsI thought the overlapping displays was part of the "random" effect you mentioned. But it sounds like you want them sequential instead, which is even easier. Just make sure that the individual action times add up to the total interval period: