Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial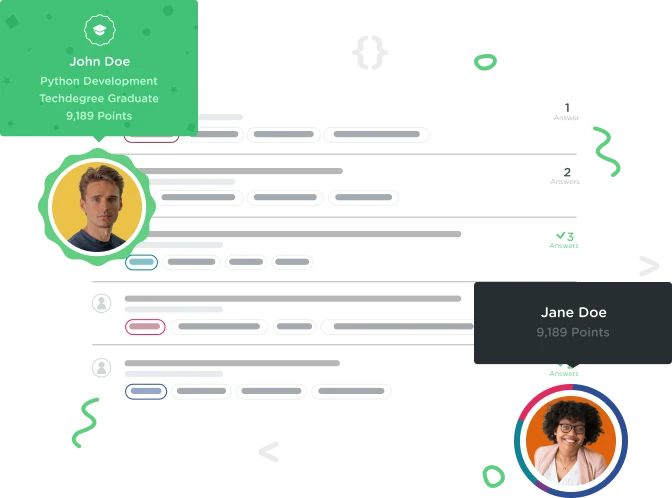
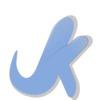
john knight
Courses Plus Student 9,155 Points2 Questions
Q1: what does the {%} mean in python || Q2: what does the [.Format] mean and do
3 Answers
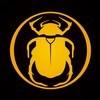
rydavim
18,814 PointsThe .format
function helps you format strings (text). The most common use case would be positional formatting. Basically, it lets you build strings with initially unknown values.
# Here you start building a string, but you don't know the user's name or number inputs yet.
# .format allows you to insert these values, given by the user.
# You want to print "Hey (name)! (\n = new line) The number (number)..."
print("Hey {}!\nThe number {}...".format(name, number))
# Example:
# Hey john knight!
# The number 10...
The %
symbol is a modulo operator. It returns the remainder of a division operation. In this case, you're using it to determine if a number is "fizz" (divisible by 3) or "buzz" (divisible by 5). Using the example above (10), it would look something like...
# Is 10 (number) divisible by 3?
is_fizz = number % 3 == 0
# 10 % 3 = 1 (remainder), so 10 is not a "fizz" number (False).
# Is 10 (number) divisible by 5?
is_buzz = number % 5 == 0
# 10 % 5 = 0 (no remainder), so 10 is a "buzz" number (True).
Hopefully that helps, but comment below if anything remains unclear. Keep up the good work!
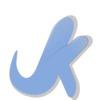
john knight
Courses Plus Student 9,155 PointsSo is there a difference if I use comma concatenation or the + symbol to add strings + a variable?
Also thank you for your responses it helped me lots!, Have a good day :D
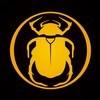
rydavim
18,814 PointsSo...that's actually kind of a tricky question. In terms of the end result of a simple use case, no there's no practical difference. But behind the scenes, or as your strings get more complicated, there are certainly advantages to using .format
.
x = "hello"
y = "world"
# Using commas (old) to print strings and variables.
# This won't work in newer versions of python, so it's commented out.
# print "Print:",
# print x,
# print y
# Output - Print: hello world (Python 2)
# Using string concatenation to construct and print a string with variables.
# NOTE: This creates the strings, then combines them together - which may not be memory-friendly in some cases.
print("Print: " + x + " " + y)
# Output - Print: hello world
# Using the format function to print strings with variables.
print("Print: {} {}".format(x, y))
# Output - Print: hello world
I would probably recommend using .format
for anything more complicated than a couple of lines or variables. Python isn't my strongest language yet, so hopefully that made sense. :)
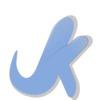
john knight
Courses Plus Student 9,155 PointsThank you very much. You just my day great! thank you.