Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial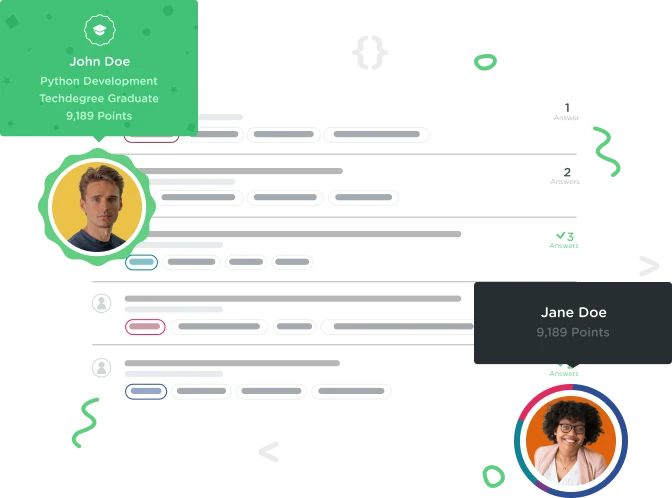

Jonathan Martínez
13,375 Points2 Questions about Rails associations!
I have 2 tables (movies and shows); movies has :name, :image and :sinopsis, shows has :time. Table 'shows' is actually referencing movies (no problem there)
## MODELS
# movie.rb
class Movie < ActiveRecord::Base
has_many :shows
end
# show.rb
class Show < ActiveRecord::Base
belongs_to :movie
end
Questions
1.- Let's say that I want to show a specific movie, and all the movie's shows. Is this the correct way of doing it?
<h1><%= @movie.name %></h1>
<%= image_tag @movie.image %>
<h3><%= @movie.sinopsis %></h3>
<table>
<thead>
<tr>
<th>Showtimes</th>
</tr>
</thead>
<tbody>
<% @movie.shows.each do |m| %>
<tr>
<td><%= m.time.strftime("%I:%M" + " %p") %></td>
</tr>
<% end %>
</tbody>
</table>
2.- Why do I have to loop through @movie.shows? Why this won't work?
@movie.shows.time
=begin
undefined method `time' for <Show::ActiveRecord_Associations_CollectionProxy:0x33919e0>
=end
Many many thanks for taking some of your time to take a look at this!
Tim Knight
28,888 PointsTim Knight
28,888 PointsJonathan,
You're definitely on the right track with how you're addressing the output in section 1. Instead of using just
m
as your index for your loop it's more common in Rails to use a word of what the index is. For example:The reason that you can't just call
@movie.shows.time
is because @movie.shows is an array of records. You'd have to select the record you want to show the times for. For example @movie.shows.first.time will return the array for the times for the first shows record.