Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial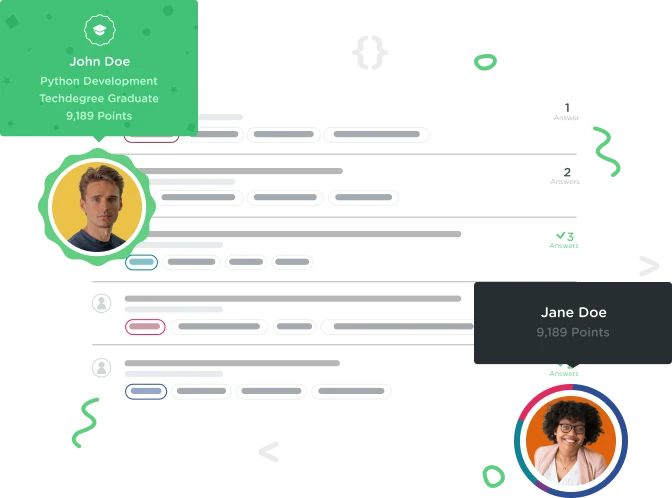
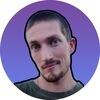
Tommy Binkley
18,584 Points2 Text boxes showing up at the same time when a button is pressed
How would I show a fact in one of the text boxes and then who created that fact in a different text box below the original fact? So every time you would push the button it would show you a different fact with the corresponding author below it.
1 Answer
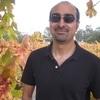
Kourosh Raeen
23,733 PointsHere's one way of doing it. Create a class that models a single fact:
package com.teamtreehouse.funfacts;
public class Fact {
private String mFactAuthor;
private String mFactText;
public Fact(String factAuthor, String factText) {
mFactAuthor = factAuthor;
mFactText = factText;
}
public String getFactAuthor() {
return mFactAuthor;
}
public String getFactText() {
return mFactText;
}
}
The above class has two member variables, one for the author of the fact and one for the fact itself. You can create a fact using the constructor that takes the author and fact as parameters, and there are a couple of getter methos too.
Next, make some changes to the FactBook class, namely change the array type from String to Fact, and then using the new operator create Fact objects and provide an author as the first parameter to the constructor. Also, Change the return type of the getFact() method from String to Fact and return the randomly selected Fact object.
package com.teamtreehouse.funfacts;
import java.util.Random;
public class FactBook {
// Fields (Member Variables) - Properties about the object
private Fact[] mFacts = {
new Fact("Joe", "Ants stretch when they wake up in the morning."),
new Fact("Bob", "Ostriches can run faster than horses."),
new Fact("Jill", "Olympic gold medals are actually made mostly of silver."),
new Fact("Jack", "You are born with 300 bones; by the time you are an adult you will have 206."),
new Fact("John", "It takes about 8 minutes for light from the Sun to reach Earth."),
new Fact("Jane", "Some bamboo plants can grow almost a meter in just one day."),
new Fact("Kate", "The state of Florida is bigger than England."),
new Fact("Bill", "Some penguins can leap 2-3 meters out of the water."),
new Fact("Meg", "On average, it takes 66 days to form a new habit."),
new Fact("Mel", "Mammoths still walked the earth when the Great Pyramid was being built.") };
// Methods - Actions the object can take
public Fact getFact() {
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(mFacts.length);
return mFacts[randomNumber];
}
}
Next, add another TextView, in the activity_fun_facts.xml file, right below the TextView for the fact. This new TextView is for displaying the author of the fact:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:id="@+id/relativeLayout"
tools:context="com.teamtreehouse.funfacts.FunFactsActivity"
android:background="#51b46d">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Did you know?"
android:textSize="24sp"
android:textColor="#80ffffff"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Ants stretch when they wake up in the morning."
android:id="@+id/factTextView"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textSize="24sp"
android:textColor="@android:color/white"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Author: Joe"
android:id="@+id/factAuthorTextView"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_below="@+id/factTextView"
android:textSize="24sp"
android:textColor="@android:color/white"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Show Another Fun Fact"
android:id="@+id/showFactButton"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:background="@android:color/white"
android:textColor="#51b46d"/>
</RelativeLayout>
Finally, make some additions and changes to the activity class. We need a new member variable for the author. Then we need to assign the corresponding TextView to that variable using the findViewById method. Then we can call the getFact() method on mFactBook, but notice that now it returns a Fact object instead of a String. We can create two local String variables for the fact and the author and assign values to them using the getter methods of the Fact class. Finally, we can set the text for the two TextViews:
package com.teamtreehouse.funfacts;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
public class FunFactsActivity extends AppCompatActivity {
public static final String TAG = FunFactsActivity.class.getSimpleName();
private FactBook mFactBook = new FactBook();
private ColorWheel mColorWheel = new ColorWheel();
// Declare our View variables
private TextView mFactTextView;
private TextView mFactAuthorTextView;
private Button mShowFactButton;
private RelativeLayout mRelativeLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Assign the Views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factTextView);
mFactAuthorTextView = (TextView) findViewById(R.id.factAuthorTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
mRelativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
Fact fact = mFactBook.getFact();
String factAuthor = fact.getFactAuthor();
String factText = fact.getFactText();
int color = mColorWheel.getColor();
//Update the screen with our dynamic fact
mFactTextView.setText(factText);
mFactAuthorTextView.setText("Author: " + factAuthor);
mRelativeLayout.setBackgroundColor(color);
mShowFactButton.setTextColor(color);
}
};
mShowFactButton.setOnClickListener(listener);
//Toast.makeText(FunFactsActivity.this, "Yay! Our Activity was created!", Toast.LENGTH_SHORT).show();
Log.d(TAG, "We're logging from the onCreate() method!");
}
}
Hope this is helpful.