Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial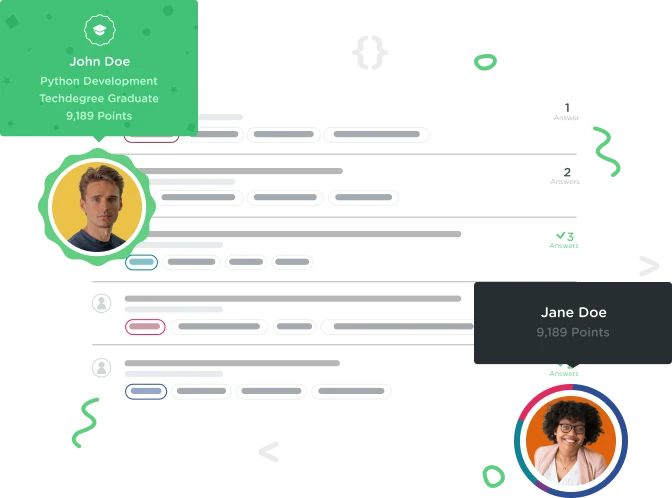

Harro Rauwerdink
4,417 Points2nd GoKart lapsDriven Overload Methods
I don't know what to do, I kinda got the first problem (still think it doesn't make much sense) but the second challenge I really don't get. Can someone please show me what I need to do? I already read the answer to someone else's question about the same thing but I'm not getting any wiser from that either.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven = 1;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(lapsDriven);
}
public void drive(int shouldBeDriven) {
lapsDriven += shouldBeDriven;
barCount -= lapsDriven;
}
}
2 Answers

Yanuar Prakoso
15,196 PointsHI Harro
You almost made it to finish the challenge. You only need to change one single line of code in your original code:
public void drive() {
//drive(lapsDriven);<--this is wrong you only need to call the other drive(int shouldBeDriven)
// but since you just want it to drive for 1 lap you must pass and integer = 1 like this:
drive(1);
}
public void drive(int shouldBeDriven) {
lapsDriven += shouldBeDriven;
barCount -= lapsDriven;
}
That should take you passing the challenge.
If you want to have longer explanation: Basically, in the start of the challenge, your drive() method should be like this:
public void drive(){
lapsDriven++;
barCount--;
}
It means that each time any user calls this method it will run (or at least simulate) the go kart for exactly one lap. Thus lapsDriven will be incremented by one (++) and barCount will be decremented by one (--). Maybe it sounds weird for many people, "Why would you only make the go kart to run just for one lap? The go Kart can runs more than one lap if the bar in the battery is still available". Well, it just that this goKart class only defines what happen if a go kart runs for a lap. Thus, it leaves the user made class that will call the method drive() inside goKart class determine how many laps it wanted to run. To do this the user made class can use for instance for loop.
However, in this challenge some users wanted us to make a drive method that can simulate what will happen when more than one lap go kart runs. That is why you make this new drive method:
public void drive(int shouldBeDriven) {
lapsDriven += shouldBeDriven;
barCount -= lapsDriven;
}
in this method your user can just pass how many laps the user wants the go kart to runs. Then the goKart class will change the state of the go kart in terms on the record how many laps it runs and how many bars left in their battery. This way users do not have to make method to define how many laps it wants the go kart to run inside their class.
However, the previous users that uses the old drive() method (please note that older drive method did not accept any argument, unlike the new one expected to accept an integer) cannot use this goKart class anymore, why? Because the method drive() is no longer exist. They demand that we as the developer of goKart class to give them their old ways of doing things, maybe because they do not want to change their code. We as developer can do this using what is called method overloading. Meaning we make more than one method with the same name but accept different arguments. This is what that last example I showed you before:
public void drive() {
drive(1);
}
public void drive(int shouldBeDriven) {
lapsDriven += shouldBeDriven;
barCount -= lapsDriven;
}
As you can see there are two drive method but the top one does not accept any arguments and the bottom one accept integer argument. Since the old style only add one lap into drive() method (please refer to the incremented and decremented explanation above) we can use this by using the other drive(int shouldBeDriven) method. We call that method from the drive() method and pass an integer of 1 to simulate the go kart runs for one lap. The result will be the same as:
lapsDriven++;
barCount--;
I hope this explanation helps and not making you more confused. Please keep on practicing and do not give up. Happy coding and good luck.

Harro Rauwerdink
4,417 PointsThank you very much for the big explanation and taking the time to look at my code. Sincerely thank you, helped me a lot!

Ken Alger
Treehouse TeacherHarro;
Method overload can indeed be confusing. In a nutshell, it allows for the creation of multiple methods with the same name that take different arguments. Let's take a look at our drive()
method here.
For the first portion of the challenge, we need to make the drive()
method accept an argument. You did a great job with this step! We could use this drive()
method to tell our application that we want to drive(5)
or drive(8)
and it will adjust the lapsDriven
and barCount
variables accordingly.
For task 2 in the challenge, we are asked to write another method called drive
. This overloads this method name and will allow us to extend some functionality. Our new drive method need to accept no arguments:
public void drive() {}
We're asked to have this new method just make one lap. We could use the code that was originally provided in the challenge:
lapsDriven++;
barCount--;
Seems pretty repetitive though, right? Since we already have a drive
method that takes arguments we could keep our code more DRY by calling that method inside our new drive
method and passing in an argument of 1
, as requested.
Think of the power of this for a moment. If, in the future, we want to add something to drive(int shouldBeDriven)
such as, I don't know, tire wear, we can do that in one place.
Post back if you have further questions and happy coding!
Ken

Harro Rauwerdink
4,417 PointsThank you for answering, helped me get further! Cheers!
Jef Davis
29,162 PointsJef Davis
29,162 PointsCan you remind me what the question is asking?