Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial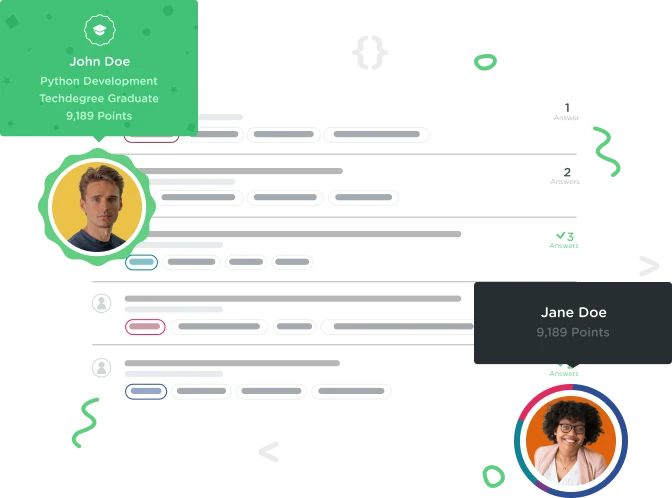

Casey Fictum
2,284 Points2nd sentence not writing to the page
I am having trouble concatenating my variables correctly. here's my code below - it works when I run like this:
var answerone = prompt('Give me a Noun');
var sentenceone = 'There once was a man ' + answerone + answertwo;
var answertwo = prompt('Give me a Verb');
var answerthree = prompt('Give me an adjective');
alert('You are done');
sentenceone += ' programmer who wanted to use JavaScript to ' + answertwo;
document.write(sentenceone);
However, it doesn't write when I add the last variable concatenation like below - --- why?
var answerone = prompt('Give me a Noun');
var sentenceone = 'There once was a man ' + answerone + answertwo;
var answertwo = prompt('Give me a Verb');
var answerthree = prompt('Give me an adjective');
alert('You are done');
sentenceone += ' programmer who wanted to use JavaScript to ' + answertwo;
sentenceone += ' at night ' + answerthree;
document.write(sentenceone);
1 Answer

Neil Docherty
10,418 PointsIt looks to me that you are making use of answertwo before you create it and assign it a value.
var answerone = prompt('Give me a Noun');
var sentenceone = 'There once was a man ' + answerone + answertwo;
var answertwo = prompt('Give me a Verb');
var answerthree = prompt('Give me an adjective');
alert('You are done');
sentenceone += ' programmer who wanted to use JavaScript to ' + answertwo;
sentenceone += ' at night ' + answerthree;
document.write(sentenceone);
Running the above code results in this:
```There once was a man NOUNundefined programmer who wanted to use JavaScript to VERB at night ADJECTIVE
In order for it to work you would have to arrange the variables like this:
```javascript
var answerone = prompt('Give me a Noun');
var answertwo = prompt('Give me a Verb');
var sentenceone = 'There once was a man ' + answerone + answertwo;
var answerthree = prompt('Give me an adjective');
alert('You are done');
sentenceone += ' programmer who wanted to use JavaScript to ' + answertwo;
sentenceone += ' at night ' + answerthree;
document.write(sentenceone);
However, you'd be as well making things a bit simpler:
var answerone = prompt('Give me a Noun');
var answertwo = prompt('Give me a Verb');
var answerthree = prompt('Give me an adjective');
alert('You are done');
var sentenceone = 'There once was a man ' + answerone + ' '+ answertwo + ' programmer who wanted to use JavaScript to ' + answertwo + ' at night ' + answerthree;
document.write(sentenceone);
I made a Codepen so you can see it in action.
Hope this helps!
Casey Fictum
2,284 PointsCasey Fictum
2,284 Pointsthank you for the catch! really appreciate it.