Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial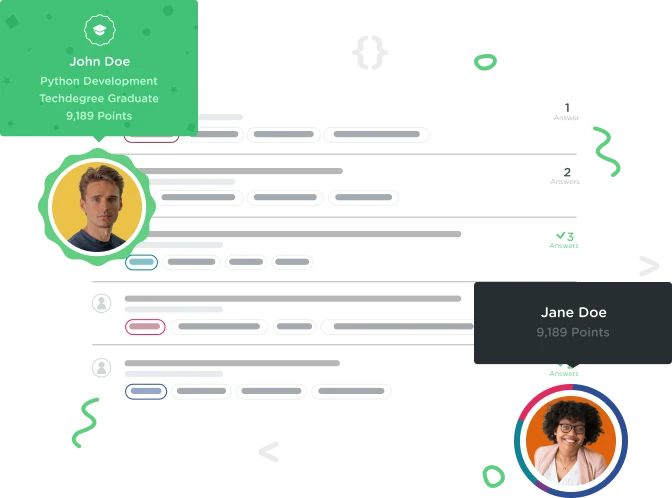
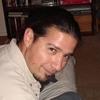
Louie Reyes
42,813 Points2nd time in two days - I'm stuck
The program runs fine on my IDE but am getting an error : java.lang.NullPointerException (Look around Prompter.java line 68) I've tried everything I can think of on line 68 but everything seems to mess up the end result. Can someone help please, I'm just not seeing it.
package com.teamtreehouse;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = "Thanks __name__ for helping me out. You are really a __adjective__ __noun__ and I owe you a __noun__.";
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.print(tmpl.render(results));
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
String inputtedWord;
System.out.printf("Please input your word for %s : ", phrase);
inputtedWord = mReader.readLine().toLowerCase().trim();
while (inputtedWord.length() == 0 || mCensoredWords.contains(inputtedWord)) {
System.out.printf("%nYou didn't input any word or it's censored, please input %s again: ", phrase);
inputtedWord = mReader.readLine().toLowerCase().trim();
}
return inputtedWord;
}
}
Please input your word for name : Louie
Please input your word for adjective : bright
Please input your word for noun : apple
Please input your word for noun : pear
Thanks louie for helping me out. You are really a bright apple and I owe you a pear.
Process finished with exit code 0
1 Answer

Yanuar Prakoso
15,196 PointsHi Louie
You need to pay more attention to the original pseudo-test.md which you should not change by the way. It just a note from Craig on what is it he really wanted you to do in the challenge. I took the liberty to go to the challenge and copy the original pseudo-test:
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story
pay attention to the pseudo-test number 1. Basically Craig wants you to ask (prompt) your user to write their own story rather than using the
String story = "Thanks __name__ for helping me out. You are really a __adjective__ __noun__ and I owe you a __noun__.";
//(this is the wrong way to do. You need to ask the user to write their own story)
that you created.
You need to create new method inside the prompter.java class to serve the user the ability to create (or in this case type) their own story using the rules you created. The rules of course each variable with __ __ will be taken as placeholders for user input.
I also took the liberty to test your code and make some changes to pass the challenge. I add this method specifically to prompt user to add their own story in the prompter.java class:
public String promptStory() throws IOException{
System.out.printf("type your story: ");
//String story = mReader.readLine();
return mReader.readLine();
}
By looking at that newly added method you can guess where to go from here right? You need to modify your Main.java class to make String story variable call that method.
Oh by the way it will be best if you also add try and catch for IOException inside your Main.java class when you call the prompter.promptStory method.
I hope this can help a little. Happy coding and good luck