Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial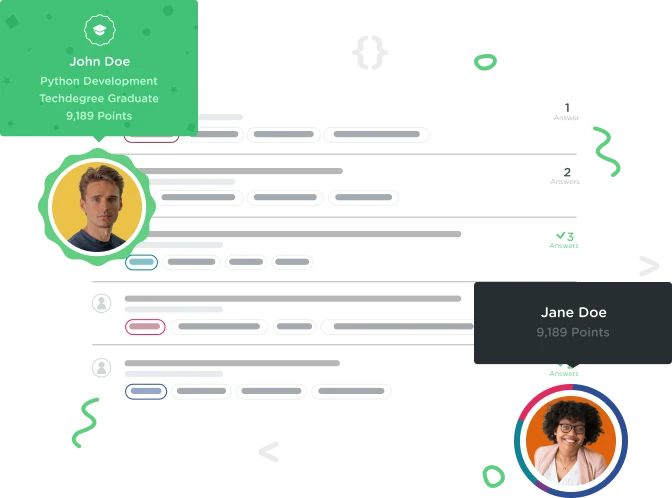

Andre Kucharzyk
4,479 Points4:54 Why we use Collection.sort(fruit) instead of....
In 4:54 why we use Collection.sort(fruit) instead of fruit.sort() or ArrayList.sort(fruit) ?
2 Answers

Yanuar Prakoso
15,196 PointsHi Andre....
Well when it comes to interface, abstract classes, classes it is when things gets complicated and scary really fast. However, in my experience learning Java, interface is very useful to set the basic rules of how classes that implements it should have as attributes of how to basically behave. However, the attributes and how to reach the goal of having such behavior is up to the class that implements it. Okay it still does not answer why don't we just write two separate class then?
Hmmmm.... let's just imagine first when you make real application you will not be working alone right? You will need support of codes from other developers. But you cannot specify down to details how the other code from other developers would be like because it will be time consumptive, not practical, and if you can do that than you better of writing the code yourself.
However, on the other hand you cannot just let other developers to put you up on any code they wish to write. If you do that there will be compatibility issues right. You need to make it clear this is what I need but how you going to achieve fulfilling my needs is up to you! Therefore, you need to make a standard on how the code should be like, just like any industry standard that define what is the specification of a product should be. Then you make it a contract to binds with other developers.
Here is where you need to make contract between you and those other developers. You need to specify the needs of your code. For instance: I need to add elements to a list, I need to be able to sort those alphabetically, and so on. But in the contract you do not specify in details how you do it. You just put some blank methods like:
interface Bicycle {
// wheel revolutions per minute
void changeCadence(int newValue);
void changeGear(int newValue);
void speedUp(int increment);
void applyBrakes(int decrement);
}
Disclaimer: I took the example from Oracle Java Docs Tutorial
As you can see inside the interface example all of the methods like changeGear, speedUp, etc. are only names with no bodies inside. However, it dictates that a Bicycle needs to be able to changeGear, speedUp, applyBrakes. Then again on how to do it and how it till behave it is up to the developers. This is what interface is all about.
I hope you are not bored yet.... Here comes the class implementing the interface, once again courtesy of Oracle Java Docs Tutorial :
class ACMEBicycle implements Bicycle {
int cadence = 0;
int speed = 0;
int gear = 1;
// The compiler will now require that methods
// changeCadence, changeGear, speedUp, and applyBrakes
// all be implemented. Compilation will fail if those
// methods are missing from this class.
void changeCadence(int newValue) {
cadence = newValue;
}
void changeGear(int newValue) {
gear = newValue;
}
void speedUp(int increment) {
speed = speed + increment;
}
void applyBrakes(int decrement) {
speed = speed - decrement;
}
void printStates() {
System.out.println("cadence:" +
cadence + " speed:" +
speed + " gear:" + gear);
}
}
That is how class implements interface, it gives the methods body to all methods name defined by the interface and able to add its' own method. You on the other hand can make your own class of Bicycle implementing the interface. Let's call you Bicycle ANDREBicycle. It was so great compared to ACME's that it can accelarate (speedUp) and decelerate (applyBrakes) faster (1.5 times faster) then you can build your own Bicycle class:
class ANDREBicycle implements Bicycle {
int cadence = 0;
int speed = 0;
int gear = 1;
// The compiler will now require that methods
// changeCadence, changeGear, speedUp, and applyBrakes
// all be implemented. Compilation will fail if those
// methods are missing from this class.
void changeCadence(int newValue) {
cadence = newValue;
}
void changeGear(int newValue) {
gear = newValue;
}
void speedUp(int increment) {
speed = speed + 1.5*increment;
}
void applyBrakes(int decrement) {
speed = speed - 1.5*decrement;
}
void printStates() {
System.out.println("cadence:" +
cadence + " speed:" +
speed + " gear:" + gear);
}
}
By doing this you already included to industry standard on how to create (instantiate) a Bicycle object. Your code should be usable if someone tries to define Bicycle and choose your model instead ACME model.
I hope I am not driving you crazy with this explanation. I am also still learning. But rest assure many people learning any programming language have episodes of difficulties understanding the concept behind every rules. How can this behave like this? Can I do this? What if I do that? Where this code coming from? That are common questions.
All I can say right now is, please keep moving forward. I know this less reassuring but as long as you let your fingers type codes your body and mind will learn and memorize them instinctively. When you get to more advanced course this question you were asked just now will be answered and sometimes without you even realizing it. Train, read, asks, exercise, and never give up. If you fail you can always try again and learn more. So please do not give up okay? Keep it up. Good luck and happy coding.

Yanuar Prakoso
15,196 PointsHi Andre....
The simplest answer is because ArrayList does not have method sort(). You can verify this in the Java documentation on ArrayList here. Therefore both fruit.sort(); and ArrayList.sort(fruit); will just not working,
Fortunately ArrayList is part of what is called Java Collection Framework as you can see in the Collection implementations table.
Therefore ArrayList object such as fruit can access the Collections Class method sort() and that is what makes Collections.sort(fruit); works.
PS: Please do not mistaken the Collections class with Collection interface
That is all from me. Additional inputs are welcome since I am also still studying here.

Andre Kucharzyk
4,479 PointsThanks for your answer.
I think the main reason for me not understanding it, is the fact that I cannot seem to grasp the idea of interfaces... I know how to implement them more or less but I don't see why would we use them since you have to basically rewrite interfaces methods...
Andre Kucharzyk
4,479 PointsAndre Kucharzyk
4,479 PointsDamn Yanuar, you are simply awesome... Every time you answer my question you give me the answer I need and understand (which is pretty unusual). Thank you for your time and patience.