Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial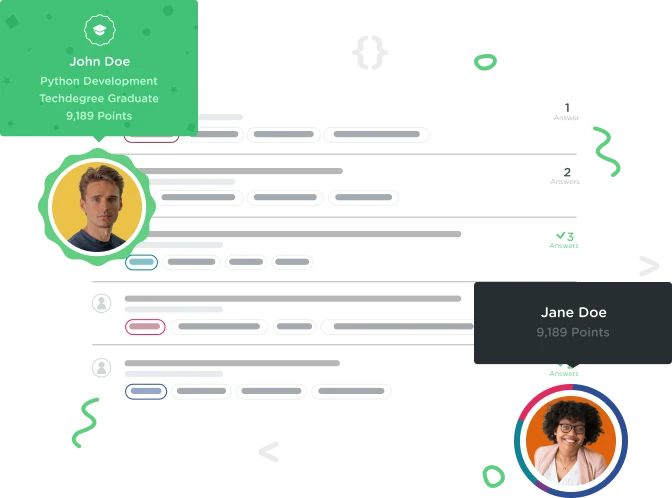

peterhampton2
2,608 Points503 Internal Error
I have no idea what is up with my spark app. I added the two extra dependencies to my gradle file in hope that it would give me a bit more information:
compile "org.slf4j:slf4j-simple:1.7.21"
compile "org.slf4j:slf4j-api:1.7.21"
But once I hit the 503 Internal Error
I get no additional information that Craig seems to be getting in this series when he comes across an error. My files look like so:
Main.java
//... imports and package declaration omitted
public class Main
{
public static void main(String[] args) {
CourseIdeaDAO dao = new SimpleCourseIdeaDAO();
staticFileLocation("/public");
port(8000);
before((request, response) -> {
if (request.cookie("username") != null) {
request.attribute("username", request.cookie("username"));
}
});
before("/ideas", ((request, response) -> {
// TODO:pjh - send message about redirect somehow
if (request.cookie("username") == null) {
response.redirect("/");
halt();
}
}));
// ... other methods omitted from this forum
get("/ideas", (request, response) -> {
Map<String, Object> model = new HashMap<>();
model.put("ideas", dao.findAll());
return new ModelAndView(model, "ideas.hbs");
}, new HandlebarsTemplateEngine());
post("/ideas", (request, response) -> {
String title = request.queryParams("title");
// TODO:pjh - This username is tied to the cookie implementation
CourseIdea courseIdea = new CourseIdea(title, request.cookie("username"));
dao.add(courseIdea);
response.redirect("/ideas");
return null;
});
}
}
CourseIdea.java
public class CourseIdea {
private String slug;
private String title;
private String creator;
private Set<String> voters;
public CourseIdea(String title, String creator) {
this.title = title;
this.creator = creator;
Slugify slugify = new Slugify();
slug = slugify.slugify(title);
}
public String getTitle() {
return title;
}
public String getCreator() {
return creator;
}
public String getSlug() { return slug; }
public boolean addVoter(String voterUserName) {
return voters.add(voterUserName);
}
public int getVoteCount() {
return voters.size();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CourseIdea that = (CourseIdea) o;
if (title != null ? !title.equals(that.title) : that.title != null) return false;
return creator != null ? creator.equals(that.creator) : that.creator == null;
}
@Override
public int hashCode() {
int result = title != null ? title.hashCode() : 0;
result = 31 * result + (creator != null ? creator.hashCode() : 0);
return result;
}
}
SimpleCourseIdeaDAO
public class SimpleCourseIdeaDAO implements CourseIdeaDAO {
private List<CourseIdea> ideas;
public SimpleCourseIdeaDAO() {
ideas = new ArrayList<>();
}
@Override
public boolean add(CourseIdea course) {
return ideas.add(course);
}
@Override
public List<CourseIdea> findAll() {
return new ArrayList<>(ideas);
}
@Override
public CourseIdea findBySlug(String slug) {
return ideas.stream()
.filter(idea -> idea.getSlug().equals(slug))
.findFirst()
.orElseThrow(NotFoundException::new);
}
}
Does anybody know why I would be receiving a 503 error? Have I set up my configuration wrongly?
2 Answers
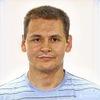
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsIt definitely does not have to do with your java files.
The best way to help you would be that you push your project on GitHub with ALL files. This is very important.
Then I can try to clone it and reproduce error.
You should also see errors in your console: something like that:
[Thread-0] INFO org.eclipse.jetty.util.log - Logging initialized @404ms
[Thread-0] INFO spark.webserver.JettySparkServer - == Spark has ignited ...
[Thread-0] INFO spark.webserver.JettySparkServer - >> Listening on 0.0.0.0:4567
[Thread-0] INFO org.eclipse.jetty.server.Server - jetty-9.3.2.v20150730
[Thread-0] INFO org.eclipse.jetty.server.ServerConnector - Started ServerConnector@73443c5b{HTTP/1.1,[http/1.1]}{0.0.0.0:4567}
[Thread-0] INFO org.eclipse.jetty.server.Server - Started @709ms
When it is a 503 error, stack trace should be printed in console.
Please attach this info as well.
One more question would be, does your App run?
What I mean by that does Spark start, and you see that App is running, or it does not?
The thing you also should do, or at least I would've done, is to clone Craigs original project:
https://github.com/treehouse-projects/java-spark-course-ideas
add your slfj
dependencies and change sourceCompatibility
in build.gradle
file
group 'com.teamtreehouse.courses'
version '1.0-SNAPSHOT'
apply plugin: 'java'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
compile 'com.sparkjava:spark-core:2.3'
compile 'com.sparkjava:spark-template-handlebars:2.3'
compile 'com.github.slugify:slugify:2.1.4'
compile "org.slf4j:slf4j-simple:1.7.21"
compile "org.slf4j:slf4j-api:1.7.21"
testCompile group: 'junit', name: 'junit', version: '4.11'
}
The app should work 100 % no 503 errors.
If it does not work, may be you somehow set up your gradle incorrectly. Then I would have checked 'introduction to Gradle` to make sure all dependencies work.
It may also be that port is busy that you are running your app into
Why do you set it to
port(8000)
Is there a reason for that ?

peterhampton2
2,608 PointsIt's weird, once I downgraded spark from 2.5 to 2.3 the error messages started showing up, and you were right about it not being a Java error. It was a handlebars error. Possibly something that hasn't been fully patched here:
https://github.com/perwendel/spark/issues/679
Thanks for your help!
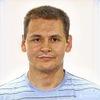
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsGreat, that you resolved it:)
Didn't help you much, but thanks for marking answer as best:) +12 points is always nice:)