Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial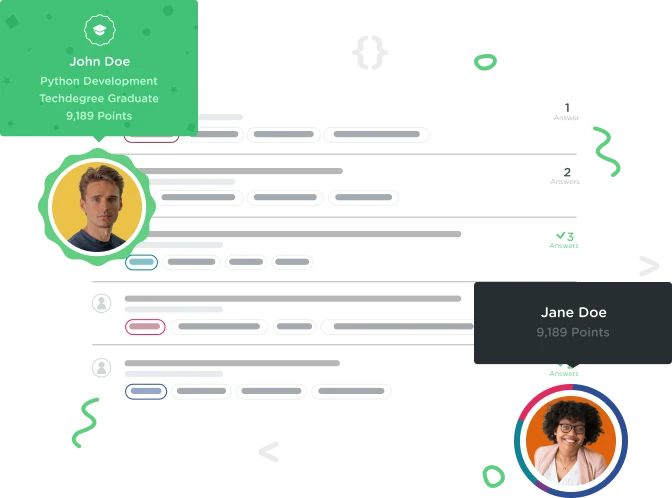
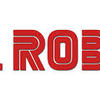
Jarvis Chandler
7,619 Points7 day forecast not working at all?
public class Day implements Parcelable { private long mTime; private String mSummary; private double mTemperatureMax; private String mIcon; private String mTimezone;
private Day(Parcelable in) {
mTime = in.describeContents();
mSummary = in.toString();
mTemperatureMax = in.describeContents();
mIcon = in.toString();
mTimezone = in.toString();
}
public static final Creator<Day> DAY_CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel in) {
return new Day();
}
@Override
public Day[] newArray(int size) {
return new Day[size];
}
};
public long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
public int getTemperatureMax() {
return (int)Math.round(mTemperatureMax);
}
public void setTemperatureMax(double temperature) {
mTemperatureMax = temperature;
}
public String getIcon() {
return mIcon;
}
public void setIcon(String icon) {
mIcon = icon;
}
public String getTimezone() {
return mTimezone;
}
public void setTimezone(String timezone) {
mTimezone = timezone;
}
public int getIconId() {
return Forecast.getIconId(mIcon);
}
public String getDayOfTheWeek() {
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
formatter.setTimeZone(TimeZone.getTimeZone(mTimezone));
Date dateTime = new Date(mTime * 1000);
return formatter.format(dateTime);
}
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel parcel, int i) {
parcel.writeLong(mTime);
parcel.writeString(mSummary);
parcel.writeDouble(mTemperatureMax);
parcel.writeString(mIcon);
parcel.writeString(mTimezone);
}
private Day(Parcel in) {
mTime = in.readLong();
mSummary = in.readString();
mTemperatureMax = in.readDouble();
mIcon = in.readString();
mTimezone = in.readString();
}
public Day() {}
public static final Creator<Day> CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel parcel) {
return new Day(parcel);
}
@Override
public Day[] newArray(int i) {
return new Day[i];
}
};
}
Taylor Bryant
7,395 PointsTaylor Bryant
7,395 PointsYou have 2 Creator methods and 2 private constructors that instantiate Days with a parcel argument. I suggest deleting one of each, when you are deleting one of the private day constructors you should delete the one that uses "describeContents()" method as you need to be using "readLong()"