Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial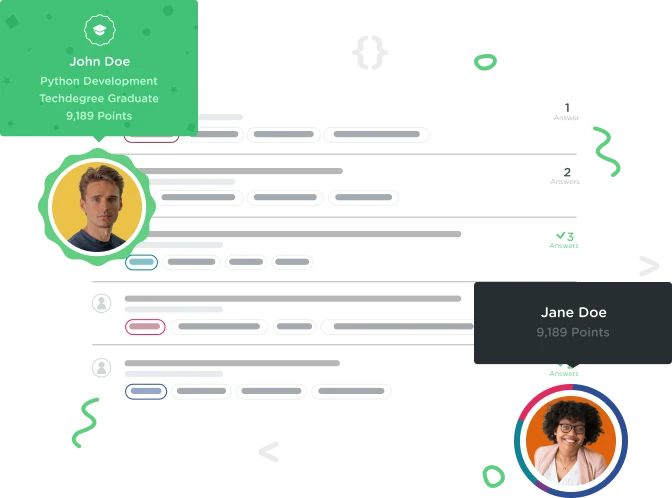

wouterb
1,307 Points7 lines of code
function rgbnr () {
var color = Math.floor(Math.random() * 256 );
return color;
}
for (counter = 0; counter < 10; counter += 1){
document.write('<div style="background-color:' + 'rgb(' + rgbnr() + ',' + rgbnr() + ',' + rgbnr() + ')' + '"></div>');
}
I have to admit that it looks a little hard to read.
6 Answers

srikarvedantam
8,369 PointsOver time, you will find it not-so-hard to read:):
- A function that generates a random intensity of color.
- A loop that writes to HTML document 10 <div>s whose background color is set to a random color (using the earlier defined function)

Nathan Crum
15,183 PointsYou can used embedded expressions to make it a little easier to read/write when creating the html string like in the code below:
var color = {'red':0,'green':0,'blue':0};
for (var i = 0; i < 10; i++) {
for (var hue in color) { color[hue] = Math.floor(Math.random() * 256 ); }
document.write(`<div style="background-color:rgb(${color['red']},${color['green']},${color['blue']})"></div>`)
}

wouterb
1,307 PointsI don't find that easier to read and write

Francisco Xifra
2,942 Pointsthis is how i did it
for (var i=0; i < 10; i += 1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
document.write('<div style="background-color:' + rgbColor + '"></div>');
}
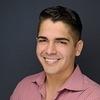
ian izaguirre
3,220 PointsJust a thought: red, green and blue are the same lines of code. So that was an area you could have reduced.

Ryan Mayo
23,604 Pointsseparating lines is optional in js:
function ran(num){ return Math.floor(Math.random() * num ); }
for(var i = 0; i < 10; i++){ document.write('<div style="background-color:rgb(' + ran(256) + ',' + ran(256) + ',' + ran(256) + ');"></div>'); }

wouterb
1,307 PointsI think line 6 is a nice example of javascript combined with html+css.

y1n5
2,626 PointsYou could just do this to further simplify (DRY the "," and calls to the function); but good job, and thanks for sharing!
function rgbColor () {
color = Math.floor(Math.random() * 256 ) + "," + Math.floor(Math.random() * 256 ) + "," + Math.floor(Math.random() * 256 );
console.log(color);
return color;
}
for (var i = 1; i <= 10; i += 1) {
document.write('<div style="background-color:' + 'rgb(' + rgbColor () + ')' + '"></div>')
}