Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial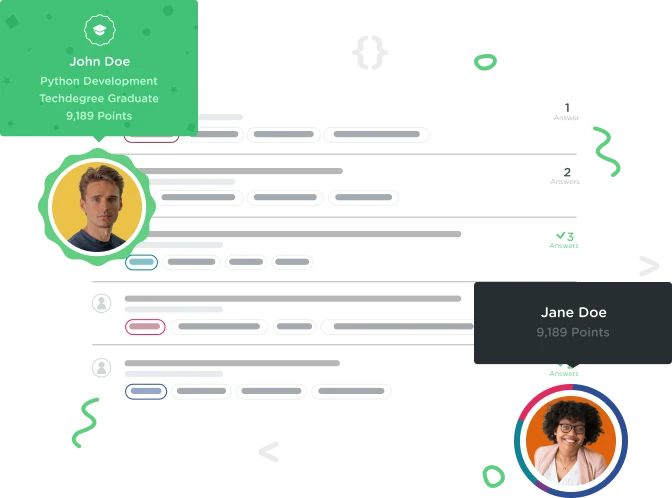

Andre Kucharzyk
4,479 Points~8:53 why we are able to add List to Set?! allHashTags.addAll(treet.getHashTags());
Why are we able to add List to a Set? treet.getHashTags() <---- List allHashTags <---- Set
for (Treet treet : treets) { allHashTags.addAll(treet.getHashTags()); <------ }
4 Answers

Yanuar Prakoso
15,196 PointsHi Andre... It's me again, I hope you are not getting bored with me... (just kidding)
Why are we able to add a List into a Set? Well the simplest answer I can give you is because they were part of Java Collection Framework. Both Set and List have the same superinterfaces: Collection<E>, Iteratable<E> (by <E> is a generic name for element of any specific object types).
But Set and List as you may have understand by watching the course video handle the collections in different manners. All classes that implement List interface allows same object to be inputted inside any kind of List more than once. Thus it will create multiple equal (true equal) objects inside a List. On the other hand Set (all kind of Set) handles collection so that no such multiple equals objects can happen. So all objects inside a Set will be unique to one another. Therefore when Craig wanted to see what hashtags is available in a tweet he uses Set because he did not need to see the same hashtags twice if the writer of that tweet accidentally doing so.
It is better if I show you with some code I guess: I wrote this code in Treehouse workspace so you can implemented it right away. It shows how we can directly transfer a List (in this case arrayList) to Sets (in this case HashSet and TreeSet):
import java.util.List;
import java.util.ArrayList;
import java.util.Set;
import java.util.HashSet;
import java.util.TreeSet;
public class TreeStory {
public static void main(String[] args) {
//now we making a list of fruits:
List<String> stringOrder = new ArrayList<>();
stringOrder.add("Guava");
stringOrder.add("Apple");
stringOrder.add("Grapes");
stringOrder.add("Banana");
stringOrder.add("Guava");
System.out.println("This is printed using ArrayList:");
for(String element : stringOrder){
System.out.printf("element: %s%n", element);
}
//now we want to convert that List (arrayList) to be a Set specifically HashSet:
Set<String> stringHash = new HashSet<>(stringOrder);
System.out.println("This is printed using HashSet:");
for(String element : stringHash){
System.out.printf("element: %s%n", element);
}
//now we use convert ArrayList into TresSet:
Set<String> stringTree = new TreeSet<>(stringOrder);
System.out.println("This is printed using TreeSet:");
for(String element : stringTree){
System.out.printf("element: %s%n", element);
}
}
}
As you can see I just directly input an ArrayList stringOrder into HashSet and TreeSet. By now I guess you also know that HashSet will only sensor the duplicated objects while TreeSet will also sort them out alphabetically. So if you compile and run that code in Treehouse workspace the result will be like this:
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
This is printed using ArrayList:
element: Guava
element: Apple
element: Grapes
element: Banana
element: Guava
This is printed using HashSet:
element: Guava
element: Apple
element: Grapes
element: Banana
This is printed using TreeSet:
element: Apple
element: Banana
element: Grapes
element: Guava
As you can see when I made ArrayList of fruits there are two "Guava"s there. But when I passed that ArrayList called stringOrder to HashSet it has no duplicated "Guava". Then when I transfer it to TreeSet I have printed all fruits in alphabetical order. I did all of that without repeating myself inputting objects over and over again to build a Set (HashSet and TreeSet). This is one of the benefit of implementing interfaces since you can implement different approach but still accessible from another class that implements the same super interface.
I hope this can help you a little. I am sorry if it just makes you even confused. But please keep on going there are still a lot ahead of you and Java is a good place to learn Object Oriented Programming. Best of luck and happy coding

Andre Kucharzyk
4,479 PointsAgain, I appreciate your help
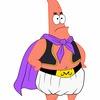
<noob />
17,062 PointsYanuar Prakoso Amazing answer, u really clarify alot of things and teached me how to convert a list into different kind of sets :D

Courtney Wilson
8,031 PointsHere's a short explanation of how I understand it: the addAll() method takes a Collection as its parameter:
addAll(Collection<? extends E> c)
Both Set and List are subinterfaces of Collection so any class that implements Set or List will inherently implement Collection. For example, HashSet implements Set so it also implements Collection. ArrayList implements List, so it also implements Collection. They can both be used as parameters for the addAll method.
https://docs.oracle.com/javase/8/docs/api/java/util/Collection.html#addAll-java.util.Collection-
Thomas Fletcher
7,284 PointsThomas Fletcher
7,284 PointsGreat explanation - I'm going back through some of the videos which i didn't completely understand and write out all of the key points. This has clarified an awful lot.
Thanks for taking the time to post.