Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial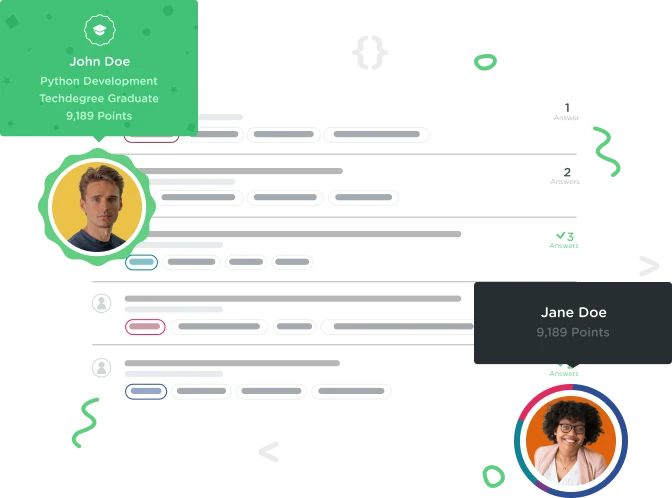

Marcatus X
834 Points99 Bottles of bears code
So I'm a Java noob and I'm learning with this book: [Head First Java][1]. I would like some help solving one exercise in the book:
public class BeerSong {
public static void main (String[] args) {
int beerNum = 99;
String word = "bottles";
while (beerNum > 0) {
if (beerNum == 1) {
word = "bottle"; // singular, as in ONE bottle.
}
System.out.println(beerNum + " " + word + " of beer on the wall");
System.out.println(beerNum + " " + word + " of beer.");
System.out.println("Take one down.");
System.out.println("Pass it around.");
beerNum = beerNum - 1;
if (beerNum > 0) {
System.out.println(beerNum + " " + word + " of beer on the wall");
} else {
System.out.println("No more bottles of beer on the wall");
} // end else
} // end while loop
} // end main method
} // end class
"There’s still one little flaw in our code. It compiles and runs, but the output isn’t 100% perfect. See if you can spot the flaw, and fix it." ??
When I ran the code in Eclipse what i could conclude is that the problem is with the spacing of the phrases(:lol what am i even saying); it does not seem to flow like a paragraph. I guess instead of using "println" we should use only "System.out.print" So what are your suggestions?
Thanks for any help
2 Answers
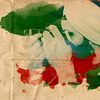
Gunjeet Hattar
14,483 PointsWell I suppose it's trying to reflect to the actual layout of the children song "99 bottles of beer" (such a thoughtful children's song :D)
So the lyrics of the song goes
99 bottles of beer on the wall, 99 bottles of beer.
Take one down and pass it around, 98 bottles of beer on the wall.
I've tweaked the program to match to this. You can easily spot the difference. Yes you were right we need to use instances of print instead of println
Modified program
public class BeerSong {
public static void main (String[] args) {
int beerNum = 99;
String word = "bottles";
while (beerNum > 0) {
if (beerNum == 1) {
word = "bottle"; // singular, as in ONE bottle.
}
System.out.print(beerNum + " " + word + " of beer on the wall");
System.out.println(", " + beerNum + " " + word + " of beer.");
System.out.print("Take one down and ");
System.out.print("pass it around, ");
beerNum = beerNum - 1;
if (beerNum > 0) {
System.out.println(beerNum + " " + word + " of beer on the wall.");
System.out.println(" ");
} else {
System.out.println("no more bottles of beer on the wall.");
} // end else
} // end while loop
} // end main method
} // end class
Hope this helps

Ho Sun Lee
2,003 PointsHi, I just wanted to throw this in there as well. I know it's late, but here it is.
This is how I did it:
package bottles;
public class Bottles {
public static void countdown(int n) {
if (n == 0) {
System.out.println("No bottles of beer on the wall, no bottles of beer, "
+ "ya’ can’t take one down, ya’ can’t pass it around, ’cause "
+ "there are no more bottles of beer on the wall!");
} else {
System.out.println((n) + " bottles of beer on the wall," + (n) + " bottles of beer, ya take one" +
"down, ya’ pass it around, " + (n-1) + " bottles of beer on the wall.");
countdown(n-1);
}
}
public static void main(String[] args) {
countdown(5);
}
}
To be honest, I'm not sure if this is the best way to do it, but this is how I ended up doing it.
Marcatus X
834 PointsMarcatus X
834 PointsThank you very much for your suggestion. I did some tweak like that on eclipse; I guess it was simple as that :)