Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial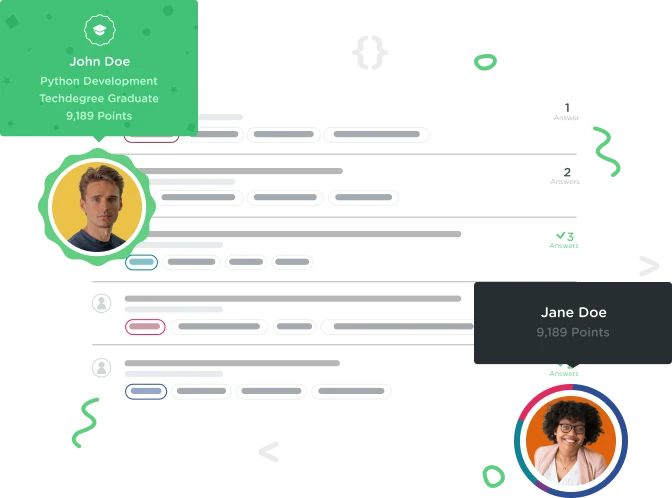

David Wilson
7,073 PointsA Better "check_speed" Method
This seems like it should work? What am I missing?
def check_speed(speed)
if speed < 45
puts "too slow"
elsif speed > 60
puts "too fast"
else speed.between(45, 60)
puts "speed OK"
end
end
4 Answers
William Li
Courses Plus Student 26,868 PointsYour code only requires a small fix to make it work. The syntax error lies on this line else speed.between(45, 60)
, as Antonio De Rose has pointed out, unlike if
& elsif
clause, else
clause does not follow up w/ a conditional check. So delete speed.between(45, 60) after the else keyword, and your code should pass.
def check_speed(speed)
if speed < 45
puts "too slow"
elsif speed > 60
puts "too fast"
else # deleted speed.between(45, 60)
puts "speed OK"
end
end
In fact, it makes perfect sense to write the code this way, because if the number is greater than 45 & less than 60, it must lie somewhere between (45, 60).

Antonio De Rose
20,885 Pointsdef check_speed(speed)
if speed < 45
puts "too slow"
elsif speed > 60 # it is a good practice, to follow in a top down approach, like
#when you check for the conditions
#first 45 then between 45 and 60 and then finally, 60 above
puts "too fast"
else speed.between(45, 60) #error, you do not have something like between with argument passed for an if else
#and else cant have a condition, it literally an else and no paranthasis with a conditon
#meaning, anything of all the above conditions, go into this scope
puts "speed OK"
end
end
#you can either have 3 separate if's, for the reason, there is nothing can be with an else condition
#or else, you can have one if and 2 elsif's which I will not encourage, as there will be an else part missing
#like the below
def check_speed(speed)
if speed < 45
puts "too slow"
end
if speed >= 45 and speed <= 60
puts "speed OK"
end
if speed > 60
puts "too fast"
end
end

David Wilson
7,073 PointsThank you both.
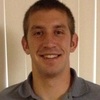
Michael Lazarz
15,135 PointsThis is what I have. In my editor it works, however in workspaces it does not. Am I missing something in translation?
def check_speed(speed)
if speed >= 60
puts "too fast"
elsif speed > 45
puts "speed OK"
else
puts "too slow"
end
end
check_speed(45) # Returns 'too slow'
check_speed(46) # Returns 'speed OK'
check_speed(60) # Returns 'too fast'