Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial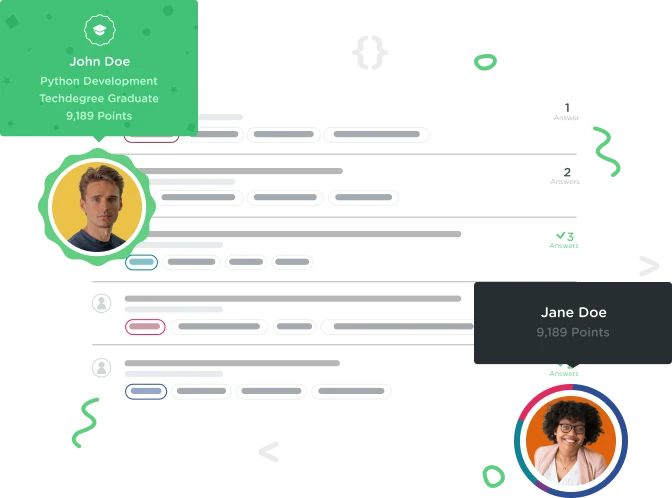
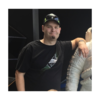
Craig Campbell
14,428 Pointsa better way to do combo() python challenge
I'm learning Python after having coded in JavaScript land. I always want to do something like this: for (var i =0; i > array.length; i++) { // some stuff}
but thats not how Python works. I solved the combo challenge in Python Collections as below, but this definitely has an un-pythonic code smell as I'm trying to use JS type thinking. While my code works, I'd like a more elegant solution. Can someone share a better way to do this?
def combo(iter1, iter2):
tup_list = []
list1 = []
list2 = []
count = 0
for thing in iter1:
list1.append(thing)
for thing in iter2:
list2.append(thing)
tup = tuple((list1[count], list2[count]))
tup_list.append(tup)
count +=1
return tup_list
3 Answers
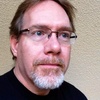
Chris Freeman
Treehouse Moderator 68,423 PointsLet's deconstruct your code:
You can create a list
from an iterable directly using list1 = list(iter1)
. but you need not create a list as you can operate directly on the iterable arguments
def combo(iter1, iter2):
tup_list = []
count = 0
for thing in iter2:
tup = tuple((iter1[count], iter2[count]))
tup_list.append(tup)
count +=1
return tup_list
You can also generate the count directly from one of the iterables:
def combo(iter1, iter2):
tup_list = []
for count in range(iter1):
tup = tuple((iter1[count], iter2[count]))
tup_list.append(tup)
return tup_list
For a more advanced solution, you can use the built-in zip
function (covered later) that "zips" two iterables together by returning one item from each iterable as a tuple. Wrapping this in a list()
provides the complete solution:
def combo(iter1, iter2):
return list(zip(iter1, iter2))
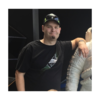
Craig Campbell
14,428 PointsThanks

Dillon Wyatt
5,990 PointsWow. That seems so much simpler than my solution.
def combo(*args):
new_list = []
counter = 0
subset1 = args[0]
subset2 = args[1]
maxer = len(subset1)
while counter < maxer:
a = subset1[counter],subset2[counter]
new_list.append(a)
counter +=1
return(new_list)
combo([1, 2, 3], 'abc')
The above example seems more straightforward than my setup using a while loop.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsEDIT: Added additional solutions.