Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial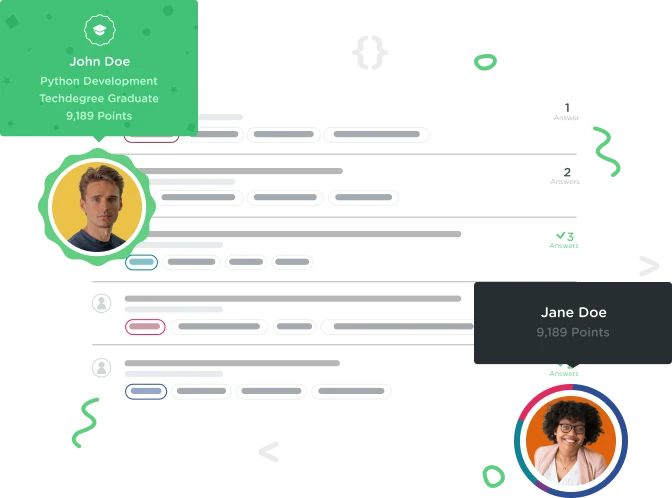

Nathalie Agnekil
6,665 PointsA bit confused about the nestled variables
I've always learned that variables is not valid outside their parent element. So how come it changes to true, when at the beginning, outside of all the nestled elements, the value is false? How can true "crawl" back up and change the false-statement?
6 Answers
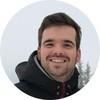
John Lack-Wilson
8,181 PointsI'm not 100% sure of what you're asking but it sounds like you're referring to scope. However, this doesn't apply here because the if/else statements within this program can freely access the variable and assign new values to it.
This would not be true if we had a program with multiple functions, where each has their own scope. This link provides quite a nice definition of both global and local scope.

Antti Lylander
9,686 Pointsvar correctGuess = false;
declares a global variable correctGuess
which can be accessed anywhere in the code below it.

Daniel Stewart
3,163 PointsScope issue Hello i may have the answer if using var the program can react in ways you would not expect. var is not locally scoped so i can update other variables with the same name either in functions or conditional statements var is hoisted meaning it can still be at the top of code block.
Solution: use Let and Const for variables.
I have studied advance scope so i not sure if this answers your questions but just highlights why us the devs don't use var

Nathalie Agnekil
6,665 PointsI'm referring to a variables lifetime. If I create or change a variable in an if-statement for example, it won't survive outside its parent element, outside the if-statement. Which means that that variable will not have been changed outside the loop. That's what I learned in C#... So I don't understand how it's been changed after the if-statement, since it's not supposed to survive outside it. It should only exist inside it.

Antti Lylander
9,686 Pointshttps://www.w3schools.com/js/js_scope.asp
As I said earlier, the variable correctGuess
in the video is of global scope and therefore can be accessed and reassigned inside a function or any statement. If you declare a variable inside a function, the variable becomes local and it does not exist outside that function.
Later on, you will learn about let
and const
that are used instead of var
nowadays. Let and const are block scoped, which I'm not going to try to explain here.

Antti Lylander
9,686 PointsIn the video, no variables are declared (created) inside if statement.

Nathalie Agnekil
6,665 PointsThe "correctGuess" variable is declared at the very top as "false", but in the first if-statement, it's value is changed to "true". And then in another if-statement, the correctGuess is used as "true" even though it's declared as "false" at the top. What I mean is that the changed value made in the first if-statement shouldn't be able to be used outside that if-statement, but in this video it IS used outside it. Do you understand what I mean?

Antti Lylander
9,686 PointsNo, I guess I don't understand what you mean. It is a global variable and there is absolutely nothing strange in the behavior of it. I copy the finished code below so you may put your comments in there. Show which part is bugging you and let's try to explain it.
/*
The Random Number Guessing Game
Generates a number between 1 and 6
and gives a player two attempts to
guess the number
*/
// assume the player didn't guess correctly
var correctGuess = false;
// generate random number from 1 to 6
var randomNumber = Math.floor(Math.random() * 6 ) + 1;
var guess = prompt('I am thinking of a number between 1 and 6. What is it?');
/* test to see if player is
1. correct
2. guessed too high
3. guess too low
*/
if (parseInt(guess) === randomNumber ) {
correctGuess = true;
} else if ( parseInt(guess) < randomNumber ) {
var guessMore = prompt('Try again. The number I am thinking of is more than ' + guess);
if (parseInt(guessMore) === randomNumber) {
correctGuess = true;
}
} else if ( parseInt(guess) > randomNumber ) {
var guessLess = prompt('Try again. The number I am thinking of is less than ' + guess);
if (parseInt(guessLess) === randomNumber) {
correctGuess = true;
}
}
//test if player is correct and output response
if ( correctGuess ) {
document.write('<p>You guessed the number!</p>');
} else {
document.write('<p>Sorry. The number was ' + randomNumber + '.</p>');
}

Nathalie Agnekil
6,665 PointsI don't know how to put my comments in....... It's this part:
First he declares correctGuess to false:
var correctGuess = false;
Then he changes the value IN an if-statement to true:
if (parseInt(guess) === randomNumber ) { correctGuess = true;
Then, in the last if-statement, he uses the variable correctGuess as true:
if ( correctGuess )
How is it possible that he can use it as true, when it's been reassigned INSIDE something else and therefore shouldn't be able to "get up" and out into the global? It should still be false since that's what it's been declared as in the global scope?

Antti Lylander
9,686 PointsNo, if a global variable is reassigned inside a function or any statement, it's value will be changed globally. At least that's how javascript works, don't know about other languages.