Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial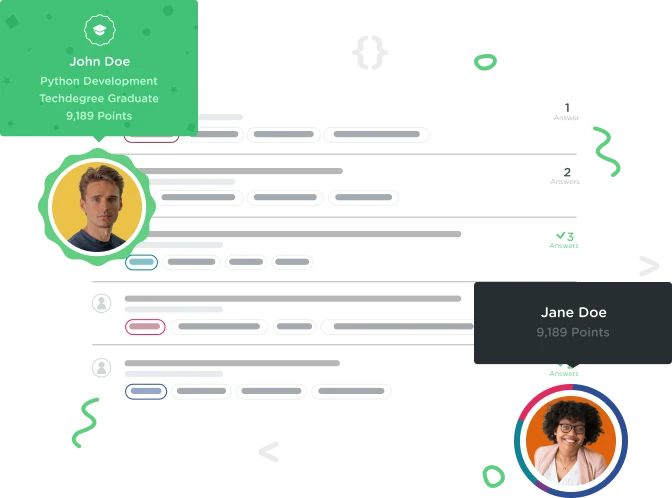
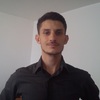
sorin vasiliu
6,228 PointsA bit stuck here, dunno how to place title above unordered list :/
So this is my code so far:
function print(message) {
var output = document.getElementById('output');
output.innerHTML = message;
}
function studentList(argument) {
var studentName = '<p>' + 'Student: ' + '</p>';
var html = '<ul>';
for (prop in students) {
studentName += '<strong>' + students[prop].name + '</strong>';
html += '<li>' + 'Track: ' + students[prop].track + '</li>';
html += '<li>' + 'Achievements: ' + students[prop].achievements + '</li>';
html += '<li>' + 'Points: ' + students[prop].points + '</li>';
}
html += '</ul>';
return studentName, html;
};
print(studentList(students));
Is it possible to place the var studentName
above each of the Name's proprieties ? I don't know how exactly to do that. Without the studentName variable
it just prints an unordered list. I want to place a "Name: + students[prop].name" above the corresponding list proprieties. And I don't know how to do that..... Is it possible or should I try to solve the challenge in another way?
Thanks !

Mario Mitchell
18,789 PointsAlso if you look at the console in Dev tools. F12 in Chrome. You'll see you have an error of 'students is not defined'. There's also an error in your return statement.
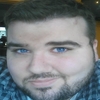
Marcus Parsons
15,719 PointsMario,
In this challenge's workspace, the students object is in a separate js file.
1 Answer
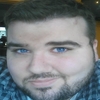
Marcus Parsons
15,719 PointsHi Sorin,
There are a few issues I'm seeing with your code, but no worries, I'm here to help you. The first issue I'm going to address is returning more than one value. In JavaScript, you can only return one item: whether that's a value, variable, object, array, etc. but it can only be one.
So, with that in mind, what I would do is instead of splitting the html you're compiling into two different variables, just keep the html variable going as it is and place the student name in a list item. Start off the html variable with that paragraph and the opening ul tag and then just add each list item to it as normal.
Also, in your argument for the studentList
function, you weren't using the argument itself. So, I changed each mention of students to argument
function print(message) {
var output = document.getElementById('output');
//changed to += so that when you do search function
//each found student data will be appended to output and not replaced
output.innerHTML += message;
}
function studentList(argument) {
var html = '<ul>';
//change all mentions of students to argument
//function was using actual students object instead of argument
for (prop in argument) {
html += '<li><strong>' + argument[prop].name + '</strong></li>';
html += '<li>' + 'Track: ' + argument[prop].track + '</li>';
html += '<li>' + 'Achievements: ' + argument[prop].achievements + '</li>';
html += '<li>' + 'Points: ' + argument[prop].points + '</li>';
}
html += '</ul>';
//you can only return one item so we return html
return html;
};
print(studentList(students));
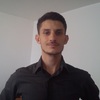
sorin vasiliu
6,228 PointsHi Marcus,
Thanks for the fast reply. I didn't post my entire code, the students
var was defined at the top. So here is the code as a whole:
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 120,
points: 14500
},
{
name: 'Sorin',
track: 'PHP Development',
achievements: 320,
points: 19000
},
{
name: 'Dean',
track: 'HTLM and CSS',
achievements: 220,
points: 15000
},
{
name: 'Mark',
track: 'iOS Development',
achievements: 190,
points: 10900
},
{
name: 'Jason',
track: 'JavaScript',
achievements: 244,
points: 305
}
];
function print(message) {
var output = document.getElementById('output');
output.innerHTML = message;
}
function studentList(argument) {
// var studentName = '<p>' + 'Student: ' + '</p>';
var html = '<ul>';
for (prop in students) {
// studentName += '<strong>' + students[prop].name + '</strong>';
html += '<li>' + 'Student: ' + students[prop].name + '</li>';
html += '<li>' + 'Track: ' + students[prop].track + '</li>';
html += '<li>' + 'Achievements: ' + students[prop].achievements + '</li>';
html += '<li>' + 'Points: ' + students[prop].points + '</li>';
}
html += '</ul>';
return html;
};
print(studentList(students));
My question is...Is there any way I can make the 'Student: ' + students[prop].name
stand out, and not be part of the UL ? Because I don't like that the whole thing is a bullet-ed list. I want 'Student: ' + students[prop].name
to be the category followed by the UL list containing Track, Achievements and Points for each Student. :P
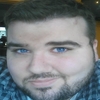
Marcus Parsons
15,719 PointsAs I mentioned in my comment, I know that the students object was there. It does not change anything about what I said above.
With that being said, why don't you just output the unordered list with a list-style of none? That way there are no bullets. The reason why you can't have that be a part of the unordered list is that you can't reprint that top statement each time. But you can modify the unordered list to look better like this:
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
function print(message) {
var output = document.getElementById('output');
//changed to += so that when you do search function
//each found student data will be appended to output and not replaced
output.innerHTML += message;
}
function studentList(argument) {
var html = '<ul style="list-style:none">';
for (prop in argument) {
html += '<li><strong>Student: ' + argument[prop].name + '</strong></li>';
html += '<li>' + 'Track: ' + argument[prop].track + '</li>';
html += '<li>' + 'Achievements: ' + argument[prop].achievements + '</li>';
html += '<li>' + 'Points: ' + argument[prop].points + '</li>';
}
html += '</ul>';
return html;
};
print(studentList(students));
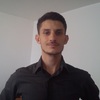
sorin vasiliu
6,228 PointsTHANKS! Everything is clear now, I understand it all and I'm ready to move to the next step! Much appreciated
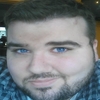
Marcus Parsons
15,719 PointsYou're welcome, Sorin. If you notice even in that 2nd piece of code I posted, I'm using the argument
variable you created for the function to call upon the students
object. It's important that you recognize that this is the way you make your function dynamic so that it can use not only the students
object but any other object that has those properties as well. There is a better way to code the function, but for right now, this will work for you.
Happy Coding, Sorin! =]
Mario Mitchell
18,789 PointsMario Mitchell
18,789 PointsHi, you should copy your code and paste it here http://jsfiddle.net and then click JSHint. You have a few errors in your syntax. Running your js through JSHInt will save you lots of time.