Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial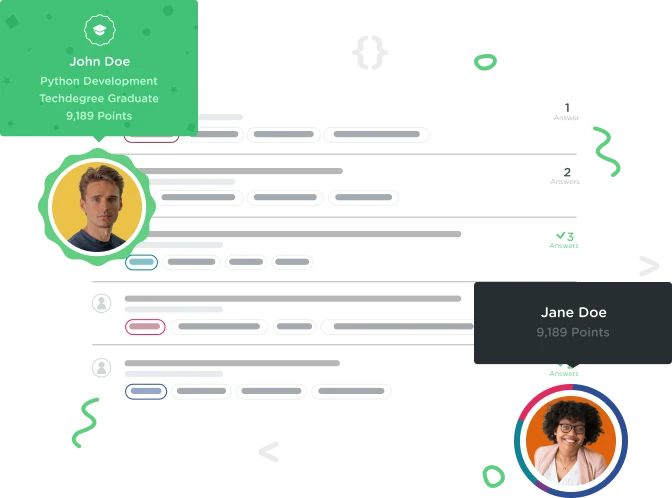

Jery Althaf
5,818 PointsA bug. I have added a constructor and it still asks if i have added it ?
public void setTopic(String topic) { mTopic = topic; }
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
public User(String firstName, String lastName) {
// TODO: Set the private fields here
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public void setTopic(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
3 Answers

Ken Alger
Treehouse TeacherJery;
I don't see a constructor in your Forum.java
class. Generally constructors take the form of:
public ClassName() {}
The task is asking us to: We are currently in the process of building a Forum. There is some skeleton code here, but I need your help to finish it up. Let's do this! In the Forum
class, add a constructor
that takes a String
for the topic and sets the private field mTopic
.
Within the constructor we need to set mTopic=topic
, assuming that topic
is the name of the String variable we have assigned our constructor to accept. The code should look something like:
public Forum(String topic) {
mTopic = topic;
}
Make sense?
Ken

Bijan Fardjad
1,775 Pointswhy is it that the challenge will accept public Forum(String topic) but not public Topic(String topic)? why does the constructor have to be named "Forum"? cant it be named anything you want? Or to make a constructor you have to have the same name as the class for it to work(can i write " for it to be referenced (work)"?)?

Jery Althaf
5,818 Pointspublic ForumPost(String topic) { mTopic = topic; }

Ken Alger
Treehouse TeacherJery;
The challenge states to build the constructor inside the Forum.java
class. Therefore our constructor needs to be in that class and have the format I mentioned above. I am not seeing the code you just posted in your original post, and specifically not in the Forum
class.
Ken

Ken Alger
Treehouse TeacherYou raise a great question about constructor methods. A constructor in java is a special type of method that is used when an object is created (initialized). It is run when the object is created, constructing the object with default values for the object.
Rules for Creating Java Constructors
To keep it simple, there are two basic rules for constructors.
- The constructor name must be the same as it's class name.
- The constructor must have no explicit return type.
Types of Java Constructors
There are two types:
- Default constructor, which takes no arguments.
- Parameterized constructor, which, you guessed it, takes parameters.
Post back if you still have questions.
Happy coding,
Ken
Francisco Navarro
14,185 PointsFrancisco Navarro
14,185 PointsHi Jery!
Could you say where exactly is the compiler warning you this?