Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial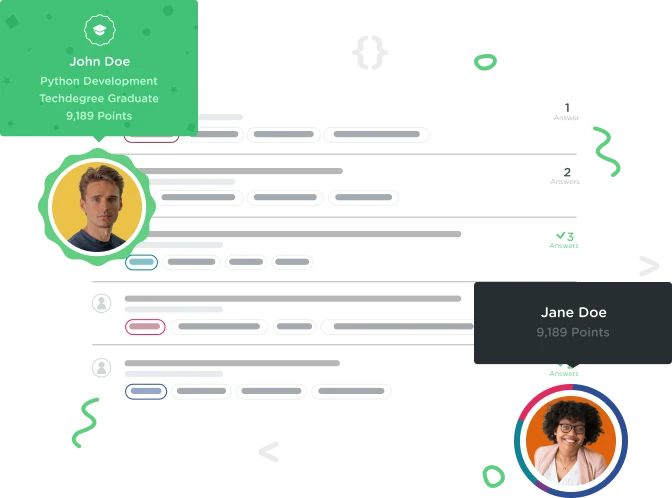

Ryan Schmelter
9,710 PointsA couple of questions of what's actually happening with this.
First, I'm hoping someone can tell me what this code is - a function? A function expression? Also, I'm wondering how this code actually works. Event (named blah here) is the parameter and we're passing the the same thing into the parameter as an argument? This confuses me because up until this point I thought of parameters as kind of placeholders for an argument. Here, it seems they need the same value.
Any explanation would be greatly appreciated.
listDiv.addEventListener('mouseover', (blah) => { if (blah.target.tagName == 'LI') { blah.target.textContent = blah.target.textContent.toUpperCase(); } });
2 Answers
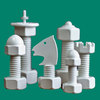
Steven Parker
229,783 PointsThat's a "function expression", which will always be the case when you create a function and pass it as an argument at the same time. The entire expression is the second argument of the call to addEventListener.
And I think your concept "of parameters as kind of placeholders for an argument" is accurate.

Stephan Olsen
6,650 PointslistDiv.addEventListener('mouseover', (blah) => {
if (blah.target.tagName == 'LI') {
blah.target.textContent = blah.target.textContent.toUpperCase();
}
});
So you're adding an event listener to the listDiv, which I asume is defined elsewhere in your code. The addEventListener takes two arguments, an event and a function. The 'mouseover' refers to the event on when the function should run. The function is an anonymous function with the ES6 arrow syntax. When an event occurs, in this case, when someone holds the mouse over listDiv, an event object is passed to the function. In your example, the "blah" parameter represents the event object. The event object is for example what you're using afterward to check if the mouseover event happened on an LI tag, and if it is an LI tag, it alters the textContent for the event.target (the list item you hovered).

Ryan Schmelter
9,710 PointsThanks, Stephen. I understand what you're saying here. However, I'm still wondering why the relationship between the parameter and the argument in this case is different than usual. For example, in this case:
function myFunction (num1, num2) { return num1 * num2; } myFunction (3, 4);
In this case, I'm passing in 3 and 4. However in the function expression, the argument and parameter are the same. I'm sure I'm just think about this the wrong way. Is this just due to the nature of an anonymous function.
Thanks for your help!
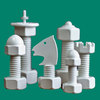
Steven Parker
229,783 PointsThis newer example is not a function expression, nor is it anonymous. It's a standard function definition followed by a call of the same function.
In the definition, the parameters are "num1" and "num2", and in the call, the arguments are 3 and 4.

Stephan Olsen
6,650 PointsI'm not quite sure I understand what you mean with the parameter and argument not being the same. However there is nothing tricky about anonymous functions, all it means is that they have no identifier/name. The example could have been written this way as well.
listDiv.addEventListener('mouseover', function(blah) {
if (blah.target.tagName == 'LI') {
blah.target.textContent = blah.target.textContent.toUpperCase();
}
});
In the example blah, represents the event object, which has properties that you can access. I'm not sure if that is what is confusing you. I made a jsfiddle with the example. When you hover an item on the list, you can see in the console the event object. It is this event object that we're using to check if the tagname is LI and to update the textContent of the event.target to be uppercase. https://jsfiddle.net/uzg63tvp/