Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial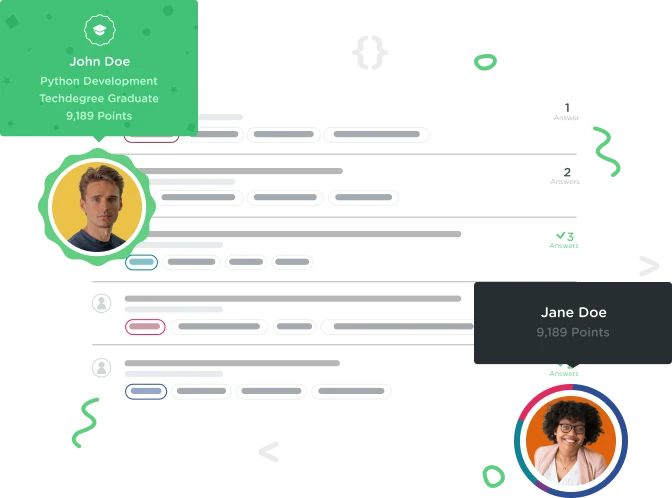
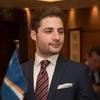
Paul Filippakis
753 PointsA different approach
What if we tried this without boolean values? They confuse me a little bit, and on my first try I did it like this. I know it works, but is it really functional? Using booleans is more of a style choice or more about a need for structured coding?
Thanks in advance, Paul
3 Answers
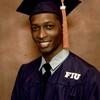
Dane Parchment
Treehouse Moderator 11,075 PointsBooleans are a fundamental datatype in Computer Science, I honestly do not believe you could write a quiz application without using them.
They are not a stylistic choice, for many applications, features, data structures, etc. they are basically required. Going out of your way to avoid using them is just going to cause more headache than necessary and you will most likely find yourself returning to booleans anyway.
In terms of booleans and your learning, what exactly is confusing? I could help you out a lot more if you let me know what is confusing about them!
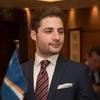
Paul Filippakis
753 PointsDear Dane, thanks for the awesome and prompt reply. Allow me to be more specific with an example. You talked about creating a quiz, and in the conditional challenge solution in JS Basics this is exactly what we did, without booleans. The following is my code - can you please elaborate on what I could replace with booleans in my code? That would be very helpful since I learn only with examples rather than explanations.
var score = 0
//Questions section
var firstAnswer = 'BITCOIN'
var firstQuestion = prompt('Which one has highest value, litecoin or bitcoin?');
if (firstQuestion.toUpperCase() === firstAnswer) {
score += 1
alert('You are correct. You have answered correctly ' + score + ' questions.');
} else {
alert('You are mistaken. You have answered correctly ' + score + ' of 5 questions.');
}
var secondAnswer = '1821'
var secondQuestion = prompt('When did the Greek Revolution began?');
if (secondAnswer === secondQuestion.toUpperCase()) {
score += 1
alert('You are correct. You have answered correctly ' + score + ' questions.');
} else {
alert('You are mistaken. You have answered correctly ' + score + ' of 5 questions.');
}
var thirdAnswer = 'STRATFOR'
var thirdQuestion = prompt('Which is the leading geopolitcal news agency for diplomatic corps?');
if (thirdAnswer === thirdQuestion.toUpperCase()) {
score += 1
alert('You are correct. You have answered correctly ' + score + ' questions.');
} else {
alert('You are mistaken. You have answered correctly ' + score + ' of 5 questions.');
}
var fourthAnswer = 'INMARSAT';
var fourthQuestion = prompt('Which is the leading satellite communications provider for the shipping industry?');
if (fourthAnswer === fourthQuestion.toUpperCase()) {
score += 1
alert('You are correct. You have answered correctly ' + score + ' questions.');
} else {
alert('You are mistaken. You have answered correctly ' + score + ' of 5 questions.');
}
var fifthAnswer = 'TREEHOUSE'
var fifthQuestion = prompt('Which is the best training platform for coding in the web?')
if (fifthAnswer === fifthQuestion.toUpperCase()) {
score += 1
alert('You are correct. You have answered correctly ' + score + ' questions.');
} else {
alert('You are mistaken. You have answered correctly ' + score + ' of 5 questions.');
}
//Rankings
if (score === 5) {
alert('Final rank: Gold Crown.')
}
else if (score === 4 || score === 3) {
alert('Final rank: Silver Crown.')
}
else if (score === 2 || score === 1) {
alert('Final rank: Bronze Crown.')
}
else {
alert('Final rank: Gold Clown. (Troll)')
}
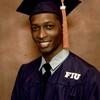
Dane Parchment
Treehouse Moderator 11,075 PointsYou don't need to add any booleans to your program, as you already have booleans in there!
Do you remember your lessons on boolean values? What do they do? Remember that a boolean is just a datatype that returns either the value True
or False
. So which statements in your program already do this?
Here is one!
(score === 5)
That is a boolean that checks if the score is equal 5 and if so returns true
otherwise returning false
. So see you have been using booleans already from the get go and haven't even realized it.
I honestly recommend that you go back and re watch every video in the section of javascript basics called: Making Decisions with Conditional Statements. Booleans are a fundamental aspect of programming and you need to be able to recognize them and recognize that you are using them.
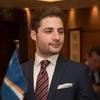
Paul Filippakis
753 PointsThank you Dane, I finally understand. I hadn't payed much attention to this section, just wanted to progress as fast as I could to get to the more juicy stuff, but it seems that patience is the way to go. thank you for your advice
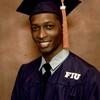
Dane Parchment
Treehouse Moderator 11,075 PointsNo problem glad to have helped. But yes, you are correct, patience is key in programming, and getting a firm understanding of the basics is an extremely important aspect of it. Remember learning a programming language is like learning a new language, and you wouldn't want to skip grammar sections of the language just to reach other parts! It is the same thing here, learning variables, datatypes, functions, etc. are key to programming, so take your time and I guarantee you that you will excel!
Paul Filippakis
753 PointsPaul Filippakis
753 PointsMy code