Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial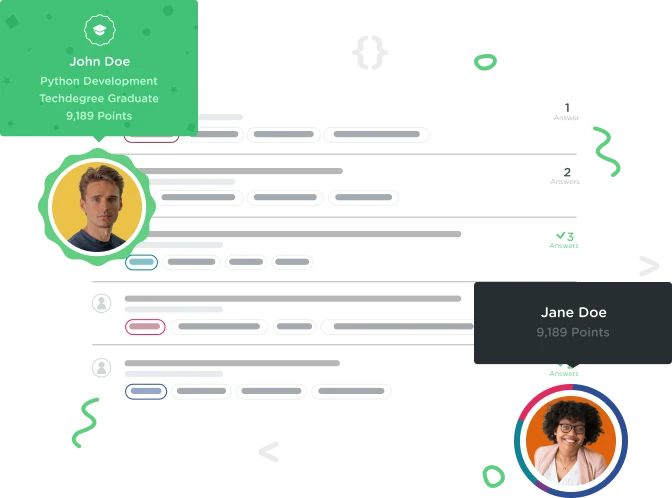

Chris Conwell
2,268 PointsA different way?
I was excited to see that My solution is almost exactly like the teacher's solution. However a minor difference. IsNaN(num1,num2) Is this proper?
// declare program variables
var num1;
var num2;
var message;
alert("Let's do some math!"); // announce the program
num1 = prompt("Please type a number"); // collect numeric input
num1 = parseFloat(num1); //convert string to float
num2 = prompt("Please type another number");
num2 = parseFloat(num2);
if ( num2 === 0) { // check for zero
alert("The second number is 0. You can't divide by zero. Reload and try again.");
}
else if (isNaN(num1,num2)){ // Is not a number?
alert("At least one of the values you typed is not a number. Reload and try again.");
} else {
// build an HTML message
message = "<h1>Math with the numbers " + num1 + " and " + num2 + "</h1>";
message += num1 + " + " + num2 + " = " + (num1 + num2);
message += "<be>";
message += num1 + " * " + num2 + " = " + (num1 * num2);
message += "<be>";
message += num1 + " / " + num2 + " = " + (num1 / num2);
message += "<be>";
message += num1 + " - " + num2 + " = " + (num1 - num2);
// write message to web page
document.write(message);
}
6 Answers
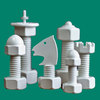
Steven Parker
231,269 PointsThe "isNaN" function only tests the first argument, the second one is ignored.
To test two arguments use the "or" operator to combine individual calls
isNaN(num1) || isNaN(num2)

Chris Conwell
2,268 PointsAhh, I see. Thank you

Sascha Laufenberg
2,961 PointsIf you do this as a recap and are familiar with functions and loops you can also combine this:
function numOne(){ num1 = prompt("Please type a number"); num1 = parseFloat(num1) if (isNaN(num1)){ numOne() } return num1 }
function numTwo (){ do { num2 = prompt("Please type another number") num2 = parseFloat(num2)} while (num2===0 || isNaN(num2)) return num2 }
numOne() numTwo()

Autumn Rhodes
925 PointsI ended up using "else if ( isNaN(num1 + num2) )", which does not seem to have been mentioned, but it works either way.
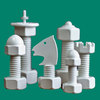
Steven Parker
231,269 PointsSince doing math on NaN is only one of several reasons an operation might produce NaN, with this test you cannot be certain that either term was NaN to begin with.

Autumn Rhodes
925 PointsI'm confused, then, Steven Parker ; when I ran the test using NaN one way or the other, it operated fine. Am I missing something?
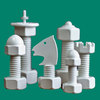
Steven Parker
231,269 PointsHere's an example:
a = "42";
b = Infinity;
console.log("a is " + (isNaN(a) ? "" : "not ") + "NaN...");
console.log("and b is " + (isNaN(b) ? "" : "not ") + "NaN...");
console.log("but a+b is " + (isNaN(a+b) ? "" : "not ") + "NaN!");
a is not NaN..
and b is not NaN..
but a+b is NaN! β![]()
This difference is writing programs that work most of the time vs. programs that work all of the time. β

Autumn Rhodes
925 PointsSteven Parker AHAH! Blind eyes regardless of surgery. Thank you!
Tural Rzazade
3,750 PointsTural Rzazade
3,750 PointsIt makes me wonder why isNaN doesn't take OR operator for two or more variables within the same parenthesesπ€
isNaN(num1 || num2)
Steven Parker
231,269 PointsSteven Parker
231,269 PointsLogical operators cannot combine terms like that, only complete expressions as in the example I gave before.
You could, however, write a function that would take any number of arguments and check if any of them were NaN:
So then you could write:
areAnyNaN(num1, num2)