Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial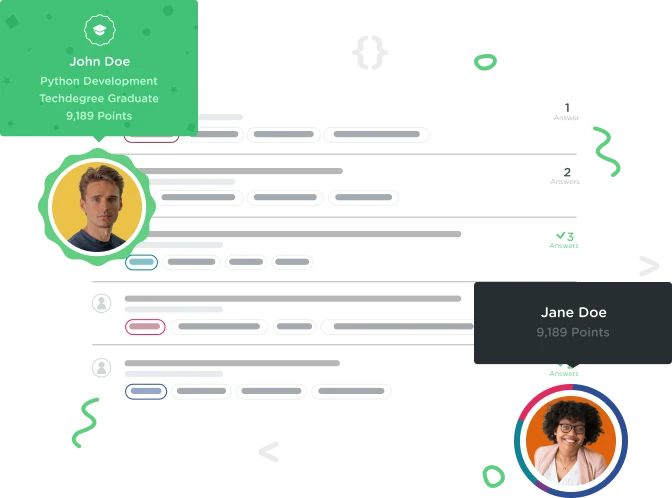

Jane Lee
1,526 PointsA few confusing points on the recap code challenge
I would just like some clarity on the following things before going forward with this module. Thanks in advance!
- Since the init method must be created 'manually' for classes, do they ALL follow the "self.__" format?
- In the last challenge, when making the switch statement cases, we have to use "self.location.y" as opposed to "location.y" - why is that? Isn't self only supposed to be used for the init method?
I feel like i'm not getting everything 100% but i'm somewhat passing the coding challenges....so a bit worrisome. Hope I can get some help better understanding these concepts. Thanks!
1 Answer
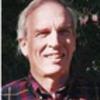
jcorum
71,830 PointsJane (1) Yes, the point of initializers are to make sure all member variables are initialized. So if they take values for those variables as parameters in the initializer, those values need to be assigned to the appropriate member variables. There are two basic ways to do this. One uses self:
init(x: Int, y: Int){
self.x = x
self.y = y
}
Here values for x and y are passed in, and assigned to member variables named x and y. self is used to disambiguate the member variable from the local variable. Some programers don't really like self (or this in other languages like Java) so they give the formal parameters different names instead:
init(ax: Int, ay: Int){
x = ax
y = ay
}
Since there's no ambiguity between the member variable x and the local variable ax self isn't needed.
(2) Think of self as a possessive pronoun: my. E.g., my x, my y You can use it anywhere you reference a member variable (in the same class). You don't need self in self.location.x, because there's no ambiguity. But it doesn't hurt if you add it. What you would be saying is "member x of my location Point variable". Here's a version (with if...else... rather than switch) without self and it works fine:
class Robot: Machine {
override func move(direction: String) {
if direction == "Up" {
location.y++
} else if direction == "Down" {
location.y--
} else if direction == "Left" {
location.x--
} else if direction == "Right" {
location.x++
}
}
}
But this version with self works just as well:
class Robot: Machine {
override func move(direction: String) {
if direction == "Up" {
self.location.y++
} else if direction == "Down" {
self.location.y--
} else if direction == "Left" {
self.location.x--
} else if direction == "Right" {
self.location.x++
}
}
}
I've seen books (for Java programming languages) that say explicitly that using this.x is very bad practice, and I've seen others that say it's the preferred or best practice! If I remember correctly I think the Apple documentation weighs in for self on the yes, use it side. Hope this helps.
Jane Lee
1,526 PointsJane Lee
1,526 PointsThanks! That definitely clarifies things a lot more!!