Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial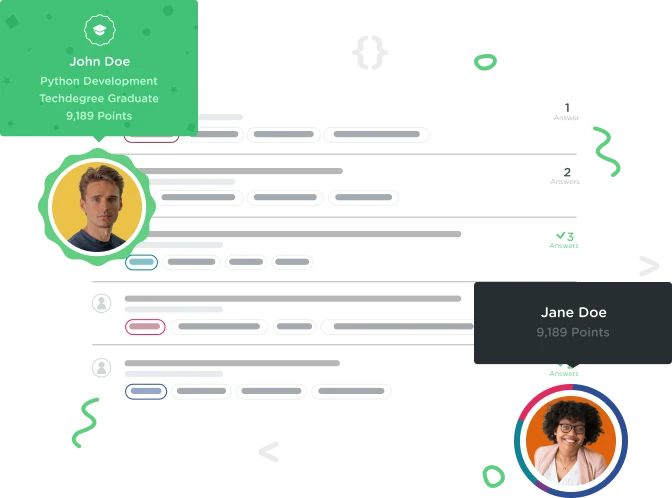
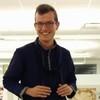
slavster
1,551 PointsA Few Optional Modifications
I wasn't a huge fan of using the pass function and thought it might be nice to give the user some feedback on why nothing happened. I modified the remove_from_list function and also created a new function called alt_show. I needed to create alt_show, as the usual show_list function began with clearing the screen, which included my message to the user. See my modifications below, and let me know if you have any feedback :)
def alt_show():
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index+=1
print("-"*10)
def remove_from_list():
show_list()
what_to_remove = input("What would you like to remove?\n> ")
try:
shopping_list.remove(what_to_remove)
except ValueError:
clear_screen()
print("I didn't see {} on the list...\n"
"".format(what_to_remove))
alt_show()
else:
show_list()
1 Answer

Holden Glass
6,077 PointsOne thing that I could see you doing is using the enumerate function on the alt_show function. The way enumerate works is in a for loop, it goes through a list and for each step it spits out two values. First is the spot is it at, the second is the value at that spot. You need to put two placeholders in your for loop to catch those values but it makes it easier to print out lists like this. I'll attach a cheat sheet.
a = ['apples', 'bananas', 'grapes', 'pears']
for spot, item in enumerate(a):
print("{}: {}".format(spot+1, item))
This should print out: 1: apples 2: bananas 3: grapes 4: pears