Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial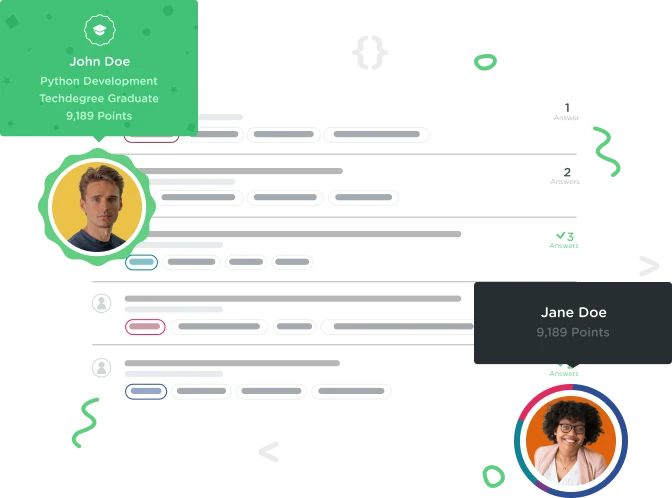
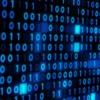
Alexander Davison
65,469 PointsA fun challenge for anybody (not only Python developers): Binary to Digits!
ALERT
This isn't a question, it's a little challenge for anybody to try.
I thought of a challenge this morning to convert binary to digits.
I have thought of converting digits to binary, but binary to digits seems easier.
If you don't know how to convert binary to numbers you can check out this video.
You actually can code this is any language, but Python is the preferred over here.
If anyone wants to try, please try to solve the problem on your own and once you've solved it, you may post your code below (optional).
I am going to post my own code in a couple programming languages including Python.
I hope you enjoy the challenge :)
~Alex
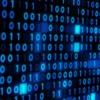
Alexander Davison
65,469 PointsAlso, if you want more challenges like this occasionally, please post below :)
5 Answers
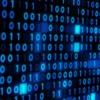
Alexander Davison
65,469 PointsMy answer in Python:
def binary_to_digit(binary_str):
result = 0
for idx, num in enumerate(binary_str[::-1]):
if num == '1':
result += 2 ** idx
elif num == '0':
pass
else:
# This line of code is just for raising an error if the string has anything but 1s and 0s
raise ValueError("Only 1s and 0s are allowed in binary")
return result
EDITED
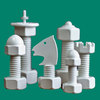
Steven Parker
231,275 PointsDid you forget to return the result?
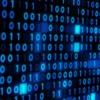
Alexander Davison
65,469 PointsOops sorry XP
I edited my answer.
I didn't copy-and-paste my code i just wrote my code in my local machine (I used IDLE) and I just tried to memorize it instead of copy-and-pasting.
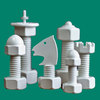
Steven Parker
231,275 Points Is it kosher to "Best Answer" yourself?
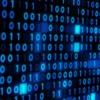
Alexander Davison
65,469 PointsMy answer in Ruby:
def binary_to_digit(binary_str)
result = 0
idx = 0
bits_reverse = binary_str.reverse.split('')
bits_reverse.each do |bit|
result += 2 ** idx if bit == '1'
idx += 1
end
return result
end
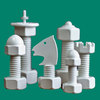
Steven Parker
231,275 PointsIn Javascript:
function binary_to_digit(binary_str)
{
let result = parseInt(binary_str, 2);
if (isNaN(result))
console.log("Only 1s and 0s are allowed in binary");
return result;
}
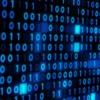
Alexander Davison
65,469 PointsHmm, I didn't know you can pass in a second parameter to JavaScript's parseInt. That's interesting! :)
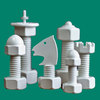
Steven Parker
231,275 PointsYes, parameter 2 (optional) is radix, I used 2 (binary) here. Very handy in this case!
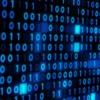
Alexander Davison
65,469 PointsAre you able to solve this task without using the parseInt
function at all? Just an additional challenge :)

Jason Anello
Courses Plus Student 94,610 PointsparseInt
is only going to return NaN
if the first character can't be converted.
So you'll get the log statement with "20" but "13" will pass through and you'll get a return value of 1.
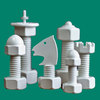
Steven Parker
231,275 PointsSure, you can do it without parseInt, just like you can check every digit for legality.
But I'm not sure I'd call either a "challenge" any more than I would apply that word to digging a 9-foot deep hole compared to digging a 2-foot deep one.
But if you like programming challenges, have you seen Code Wars?

Dave Laffan
4,604 PointsOk, I got this. The only thing I can say with confidence is I know there will be a million better and more efficient ways of doing it, but I'm happy with that because I'm new to all this and I did it based only on what I've learned so far
def binary_to_digits(arg1):
binary_list = list(arg1)
count = 1
decimal = 0
for each_entry in reversed(binary_list):
if each_entry == '1':
decimal = decimal + count
count += count
print(decimal)
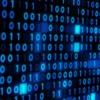
Alexander Davison
65,469 PointsGood job! No, I can't think of a way to do this a million times better. There might be simpler ways, but this is probably the cleanest way to do it out of all of the ways I can think of :)
Congrats!

Dave Laffan
4,604 PointsOh, cool, thanks!
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsNo peeking until you complete the challenge :)