Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial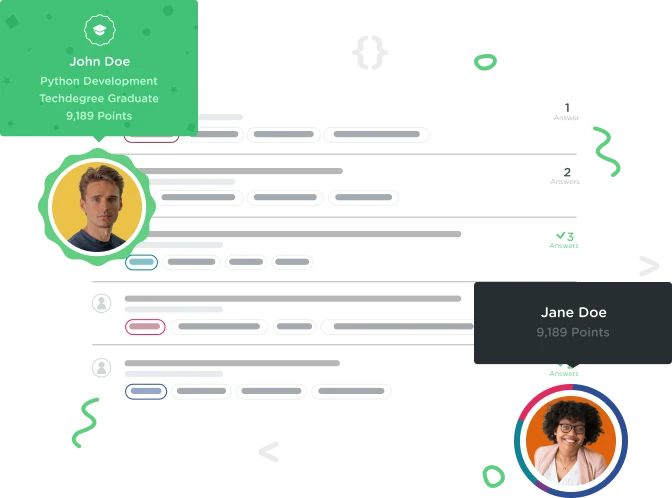
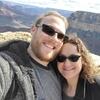
James Crosslin
Full Stack JavaScript Techdegree Graduate 16,882 PointsA little cleaner solution
I feel like this solution is a bit cleaner than Andrews, and with an added bonus, I've thrown in a try...catch
in case the user gets silly about their URIs in the /cards
route. Let me know what you think and if you have any suggestions!
cards.js
const express = require("express")
const router = express.Router()
const {
data: { cards }
} = require("../data/flashcardData.json")
router.get("/", (req, res) => {
let id = Math.floor(Math.random() * cards.length)
res.redirect(`/cards/${id}`)
})
router.get("/:id", (req, res) => {
try {
let { side } = req.query
const { id } = req.params
if (!side || side.toLowerCase() !== "answer") {
side = "question"
}
res.locals = {
name: req.cookies.username,
id,
side,
text: cards[id][side],
hint: cards[id].hint
}
res.render(`card`)
} catch (err) {
console.error(err.message)
res.redirect("/cards")
}
})
module.exports = router
card.pug
extends layout
block content
section#content
h2= text
if side === 'question'
p
i Hint: #{hint}
a(href=`${id}?side=answer`) Answer
else
a(href=`${id}`) Question
br
a(href='/cards') Random Card
1 Answer

Mike Straw
7,100 PointsI like that solution as it treats an error as an error.
I took a different approach to get the same thing by moving the redirect into a general else
statement, so if anything besides side=question
or side=answer
is there, it redirects to the question.
In the interest of DRY I also broke out the render functionality into a separate function. (I made it a const
function so all the data didn't need to be passed as parameters.
const express = require('express');
const router = new express.Router();
const {data } = require ('../data/flashCardData.json');
const { cards } = data;
router.get('/', ( req, res) => {
const id = Math.floor(Math.random() * cards.length);
res.redirect(`/cards/${id}`);
});
router.get('/:id', (req, res) => {
const { side } = req.query;
const { id } = req.params;
const text = cards[id][side];
const { hint } = cards[id];
const templateData = {
text,
side
};
const renderCard = ( flipSide ) => {
templateData.flip = flipSide;
res.render('card', templateData );
};
if ( side === 'question' ) {
templateData.hint = hint;
renderCard( 'answer' );
} else if ( side === 'answer' ) {
renderCard( 'question' );
} else {
res.redirect('?side=question');
}
});
module.exports = router;
James Crosslin
Full Stack JavaScript Techdegree Graduate 16,882 PointsJames Crosslin
Full Stack JavaScript Techdegree Graduate 16,882 PointsI really like how you broke out the render into its own expression! I'll have to remember that for later!