Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial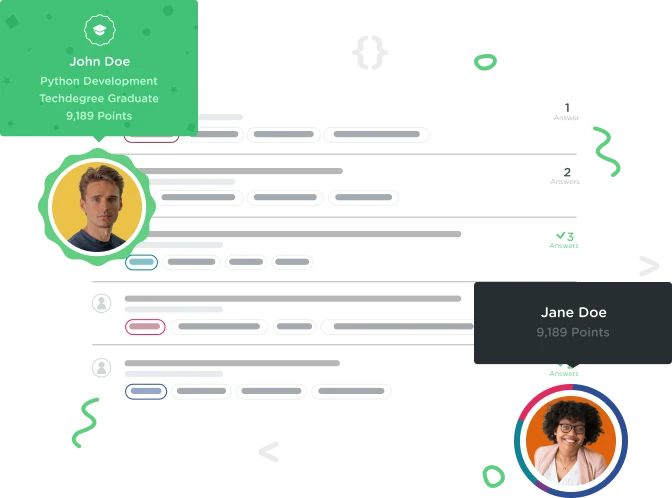

Aditya Puri
1,080 PointsA little confused..
So I have some questions....
1) Is a type of exception, like the "IllegalArgumentException" a class? Is that why he wrote throw new IllegalArgumentException
?
2) Why did he write IllegalArgumentException iae
on the 31st line of Example.java.
2 Answers

andren
28,558 PointsYes, exceptions in Java are classes.
When an exception is thrown an instance of the exception is created (just like you create instances of your own classes) the parameter list of the catch block is somewhat like the parameter list of a method, he is simply saying that the block of code requires an object of type IllegalArgumentException and that it should be set to the variable iae within the code block.
So when he calls iae.getMessage() on line 33 he is calling the getMessage method found on the IllegalArgumentException object that was created when the exception was thrown.
Exceptions are probably a bit confusing at this point but as you learn more about classes and inheritance and other such concepts, I'm sure you'll come to understand how they work. Heck you can even create your own exceptions once you learn a bit more about those things.

Aditya Puri
1,080 PointsAditya Puri
1,080 Pointsoh!! Thx for your answer! Really helped!
BTW about the second one, it stores the instance of the class "IllegalArgumentException" thrown in the class PezDispenser in a variable called "iae". And we didn't name that instance and just stored it in a variable called "iae"?
andren
28,558 Pointsandren
28,558 PointsYes, "throw new IllegalArgumentException" create a new instance of the IllegalArgumentException and immediately passes it back to the method that called the method the exception was thrown in, Java will then look for a catch block that accepts objects of type IllegalArgumentException, once it finds one it passes it to the code block.
So a catch block like this: catch(IllegalArgumentException iae) is essentially saying, run me if the exception is of type IllegalArgumentException, and set it to a variable called iae inside of the catch block.
Do note that i'm simplifying things somewhat in this explanation, I'm intentionally avoiding getting too much into class inheritance for example, which plays a somewhat important role in understanding exception management, in order to keep it less confusing.
But hopefully this explanation makes you feel somewhat comfortable using and handling exceptions in your own code.
Aditya Puri
1,080 PointsAditya Puri
1,080 PointsOh, 1) But I didn't fully understand this part
and immediately passes it back to the method that called the method the exception was thrown in
2) What if there are more than 1 catch blocks that accept objects of type IllegalArgumentException?
3)Why do we use try, catch blocks? Can't we just throw the exception? It shows the warning too right?
Sry for asking too many question tho..:D
andren
28,558 Pointsandren
28,558 PointsThink of it like a reverse method call, when you call a method that takes an argument you pass an object to the method, a throw basically does the opposite, it takes an exception object and hands it back to the method that called it, and then hands it to the catch block setup to catch it.
Java will not compile if you have a catch clause that catches an error that will be caught by an earlier catch clause.
Not catching the exception leads to the program crashing, when you catch an exception you can write some code to handle that error and then resume execution of the program.
Aditya Puri
1,080 PointsAditya Puri
1,080 Pointsabout the 2nd, can't there be 2 methods and both of them has a IllegalArgumentException? What will we do in that case if we can only write 1 exception of this type?
andren
28,558 Pointsandren
28,558 PointsYou can only have 1 catch block for each exception type per try block, if you have two methods you want to test for a certain exception and want different code to run based on which method failed then you'll simply have to place them in separate try...catch blocks like this:
That would be valid, when I said you could not have two catch blocks for the same exception I meant a setup like this:
The above example would not compile, and even if it did the second catch block would never run since the IllegalArgumentException would always be caught by the first catch block.
I realize I never explicitly mentioned the fact that you can have multiple catch blocks underneath a try block, but that is indeed possible, and is what I meant to refer to in my previous comment, it should also be noted that once a method throws an exception inside of a try block none of the remaining code in the try block is executed, so you'll never have more than one exception being thrown from a try block.
Aditya Sheode
1,291 PointsAditya Sheode
1,291 PointsDoes that mean you have to explicitly throw and specify the exception before you catch it first? like throw first and then use it in the catch block?