Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial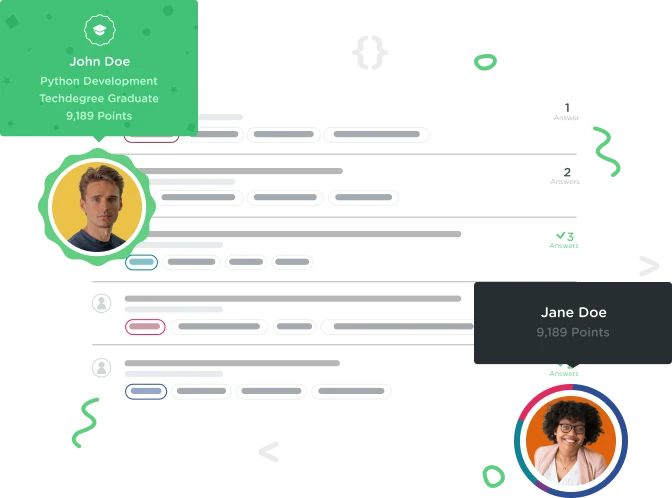

Daniel Diemer
1,937 PointsA little help understanding parameters used in other classes.
In the video, we are creating a method public bool OnMap(Point point)
Jeremy then sets up a bool inBounds to return a statement based on X Y values in the Point class.
My question is regarding using those X and Y values if it wasn't inside another method?
For example, if it was not inside that method, would we have to call it using Point.X instead of point.X? Since we already called it in the initial method, and reassigned it to point, that allows us to use the lower case point, is that at all correct?
Why do we even have to make a second lower case point in the first case?
Instead of:
public bool OnMap(Point point)
{
bool inBounds = point.X >= 0 && point.X < Width && point.Y >= 0 && point.Y < Height;
}
Why cannot we just do this?
public bool OnMap(Point)
{
bool inBounds = Point.X >= 0 && Point.X < Width && Point.Y >= 0 && Point.Y < Height;
}
Thanks, I'm just trying to fully grasp what is going on here.
2 Answers

John Thompson
4,729 PointsZ is not a attribute of the Point class. You need to write code to make it part of the Point class. As to your confusion part, what will happen is when you go back to class Game you will create a method that will trigger when a person clicks on part of the defined map, that will then activate and create a Point class and log the spot. Then you will write map.OnMap() and place the variable that was assigned to the Point class that was created when you clicked a point on the map, into the () and the method will run the code and return whatever it needs. So, basically yes. We are only able to use it because, we specified that this function will take in a Point class.
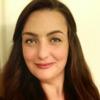
Jennifer Nordell
Treehouse TeacherHi there! You can think of the parameter as a variable declaration that will only be used in that particular method. Once the method ceases execution that variable name will disappear. The uppercase Point
is the type of the variable. The lowercase point
is the name of the variable. We could have named that point
just about anything in reality. But we like to give meaningful names. So that same code could look like this:
public bool OnMap(Point yourPoint)
{
bool inBounds = yourPoint.X >= 0 && yourPoint.X < Width && yourPoint.Y >= 0 && yourPoint.Y < Height;
}
Again, the Point
is simply declaring the type of the variable. In this case, it's a custom made type by your custom class as opposed to String
or int
or one of the built-in data types. The lower case point
/yourPoint
is the variable name we're giving to the data we just sent in.
Hope this helps!

Daniel Diemer
1,937 PointsI think I understand, but want to ask a follow up question to clarify.
Say in your example, we wanted to make the bool inBounds true or false based not only on XY coordinates, but also taking something from another type we have created, say something from the Tower.cs sheet we created earlier.
Lets say we set up the Tower with a Z coordinate and want to return true for bool inBounds only when Z == 0, to verify that the point is on the floor of a 3D map.
could I just add another statement at the end, so it would look like the following, using your example?
bool inBounds = yourPoint.X >= 0 && yourPoint.X < Width && yourPoint.Y >= 0 && yourPoint.Y < Height && yourPoint.Z = 0;
Or would that not be right since Z is a different type, Tower, not Point, and in the original method, we only defined the Point type.
Or would the Z value still work because it is a public variable?
This is the part I'm a little confused about. Are we allowed to use X and Y in the method statement only because we used the Point type in the original bool OnMap(Point yourPoint) statement?