Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial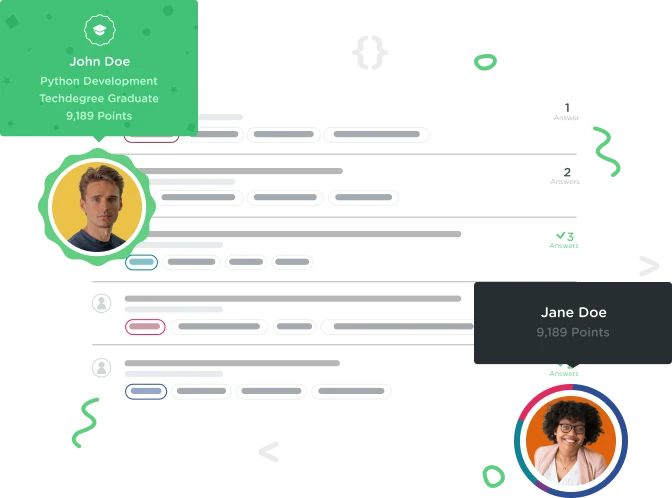
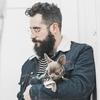
Alexander D
8,685 PointsA little TEST with a LOOP. System says there's an error, but it works!
I'd love to have a second pair of eyes on this test, as I am getting an error message from the system. I need to re-write this with a DO-WHILE statement. The original piece is this below:
var secret = prompt("What is the secret password?");
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
This is what I wrote, and it's actually working. You can even remove the variable and state the password directly into the loop.
var password = "sesame"
do {
var secret = prompt("What is the secret password?");
if (secret === password) {
}
} while (secret !== password)
document.write("You know the secret password. Welcome.");
When I paste this into the exercise below, I am getting the error message:
"Bummer: You should declare the secret
variable before the loop. Otherwise, you re-create that variable each time through the loop."
The exercise is the following:
From what I see, the program runs smoothly, so I am not too concerned. Is there any syntax error that can be avoided?
Thanks!
var secret = prompt("What is the secret password?");
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

Michael Jäkel
6,373 PointsHi Alexander, may be, it is just this little change to declare the variable secret before the loop. So the code might look like this:
const password = "sesame"
var secret = ""
do
{
secret = prompt("What is the secret password?");
if (secret === password)
{
}
} while (secret !== password)
document.write("You know the secret password. Welcome.");
The variable is declared once and used many time. Will you give this a try in your challenge?
Note: I also changed
var password = "sesame"
to
const password = "sesame"
because the password is constant and will not being changed (in your code).
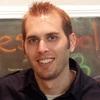
Rick Buffington
8,146 PointsI think you are confusing the instructions. They want you to declare the SECRET variable before the loop. ;)
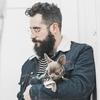
Alexander D
8,685 PointsThanks Rick! I didn't know to declare the variable separately from the prompt command. The solution has been posted below and it worked. Thanks for your answer!
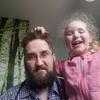
Peter Smart
9,150 PointsI've come across the same problem, for apparently the same reason. Perhaps the problem could be presented better.
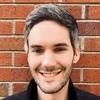
Jesse Vorvick
6,047 PointsThe error message in the challenge has nothing to do with whether the program works or not, it is saying that you want to declare the variable before the loop instead of in the loop, because otherwise you are literally recreating the variable each time the loop runs. This might not be noticeable in such a small-scale example, but I think the habit should be established now to avoid any unforeseen complications in more complex code. So while your code is grammatically correct, it is considered poor form in this situation to not declare the variable before the loop.
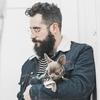
Alexander D
8,685 PointsThanks for your response Jesse! It's definitely good practice to declare the variable at the top, I'll keep that in mind!
Alexander D
8,685 PointsAlexander D
8,685 PointsThanks Michael,
That is insane!
The editor accepted the program with no problems and I passed the challenge! I am just surprised that it's possible to declare an empty variable such as 'var secret = " " '. I'll keep that in mind!