Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial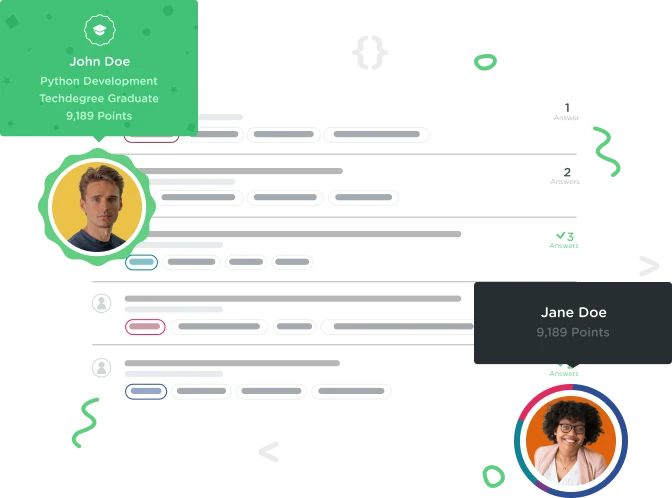
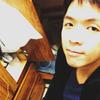
Roy Huang
4,367 PointsA lot of questions about classes, class variable and instance variable.
Hello, I found that I have trouble understanding these stuff.
What the purpose of use classes?
Why do we need a def initialize(name)? Does the [initialize] can be replaced by anything I want?
what the purpose of set variable "bank_account = BankAccount.new(name)"?
What the difference between class variable (@@var) and instance variable(@var)?
There are so many questions but I really felt lost.
Thank you very much.
2 Answers
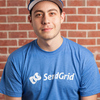
Dylan Shine
17,565 PointsClasses are used to define a "class" of objects you will use in your program. For example, if I wanted to create a program that had dog objects, I would want to make a Dog class.
class Dog
end
Think of the Dog class as a base for all of my dog objects. In order to create a new dog, I need to instantiate it from my Dog class.
In order to do so, we add an initialize method to our class. What the initialize method does is sets default attributes to our new dog object when we create it.
class Dog
def initialize(name)
@name = name
end
end
spot = Dog.new("Spot")
You cannot substitute the name of the initialize method. I create a new Dog object by assigning a variable, spot, to the Dog.new("Spot") method call. As you can see I added a parameter to my initialize method, name, and then assigned it to an instance variable @name. Instance variables store data particular to a specific instance/object of a class.
Lets say I had two dogs:
class Dog
attr_reader :name
def initialize(name)
@name = name
end
end
spot = Dog.new("Spot")
rufus = Dog.new("Rufus")
spot.name => "Spot"
rufus.name => "Rufus"
Even though both the spot and rufus Dog objects share the same instance variable, name, "Spot" and "Rufus" are unique to them because of how they were created (by passing their name as a string when calling Dog.new). You may notice I added the attr_reader method at the top of the Dog class, I suggest you look up what that does as it is a very helpful ;).
Finally class variables. As a Rubyist I avoid using them as they hold a quasi global-esque scope within our classes, that as object oriented programmers we try to avoid, but for educational purposes I'll explain.
Lets say we wanted to share a number across all of our objects in a class, for instance a num of times a method is called. First we can create a class, set a class variable count to equal 0, and create a method that increments that count by 1 every time it's called.
class SomeClass
@@number_of_times_method_is_called = 0
def what_is_the_number_of_times_the_method_has_been_called
puts @@number_of_times_method_is_called
end
def add_one_to_our_ridiculously_named_class_variable
@@number_of_times_method_is_called += 1
end
end
someClass1 = SomeClass.new
someClass2 = SomeClass.new
someClass1.count => 0
someClass2.count => 0
someClass1.add_to_count => 1
someClass2.counter => 1
Notice that when I added 1 from the someClass1 object, the count was also changed for the someClass2 object. This is because the data isn't stored within an individual object like an instance variable, but within the class as a whole. When this is the case, all of the instances of that class will have access to that same class variable, if I manipulate with one instantiated class object, it will affect it for the rest of them.
I hope this helps!

Dawa Sherpa
1,816 PointsGreat explanation of attr_reader method here: http://stackoverflow.com/questions/4370960/what-is-attr-accessor-in-ruby
Roy Huang
4,367 PointsRoy Huang
4,367 PointsHello, Dylan, Thank you very much! This is a very clear and helpful explanation !