Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial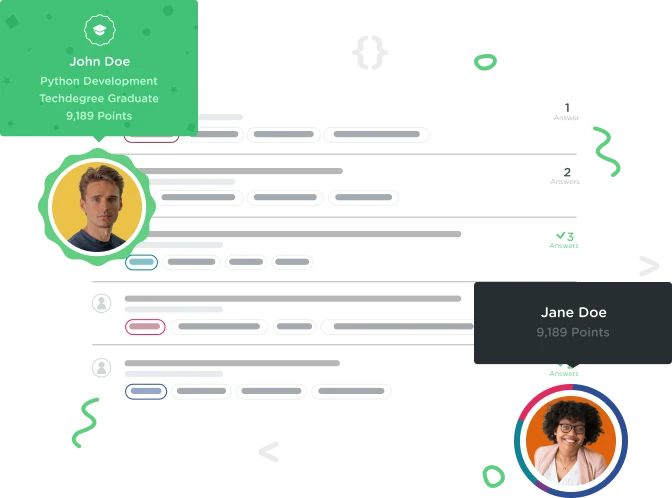
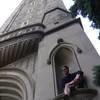
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsA nice little module for outputting a message to the console while server running
I wrote a little module that will display a nice animated message to the console while your server is running. The animation is helpful in letting you know everything is running well.
Module:
/**
* @param portNum - port server is listening on
* @param speed - interval to run the animation on (500 recommended)
*/
module.exports = function runConsoleMsg(portNum, speed) {
console.log(`Node server listening on port ${portNum}`);
let dot = '';
const message = `Node server listening on port ${portNum}`;
setInterval(() => {
console.clear();
console.log(message + dot);
dot.length > 2 ? dot = '' : dot += '.';
}, speed);
}
Put this module in a lib
folder and then require it in your index/app.js file:
const runConsoleMsg = require('./lib/[filename].js');
Then call the function in the app.listen
callback:
app.listen(8000, () => runConsoleMsg(8000, 500));
Please feel free to use and share.
2 Answers
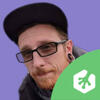
Travis Alstrand
Treehouse TeacherThanks for sharing!!

JASON LEE
17,351 PointsI tried your code. It's pretty cool. My only suggestion is to edit the console.clear()
part such that the only thing that clears is the 3 dots. In other words it doesn't clear the entire console. I don't know how to accomplish this though or if it's even possible.