Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial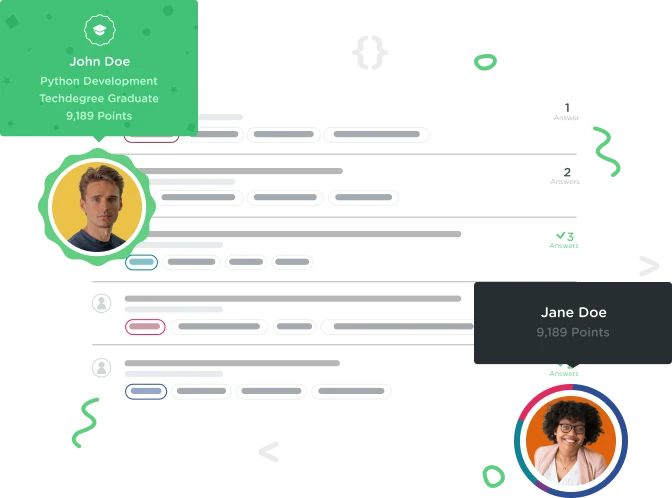

Thomas Katalenas
11,033 Pointsa != nil ? a! : b a != nil ? a! : b a != nil ? a! : b a != nil ? a!
a != nil ? a! : b from this webpage at the bottom of the video
The nil coalescing operator (a ?? b) unwraps an optional a if it contains a value, or returns a default value b if a is nil. The expression a is always of an optional type. The expression b must match the type that is stored inside a.
The nil coalescing operator is shorthand for the code below:
a != nil ? a! : b
The code above uses the ternary conditional operator and forced unwrapping (a!) to access the value wrapped inside a when a is not nil, and to return b otherwise. The nil coalescing operator provides a more elegant way to encapsulate this conditional checking and unwrapping in a concise and readable form.
Note
If the value of a is non-nil, the value of b is not evaluated. This is known as short-circuit evaluation.
here we go so the value of a is non-nil would be a != nil,
what is " a! "mean? and why the colon before b " :b "
a != nil ? a!: b
1 Answer
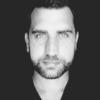
Jhoan Arango
14,575 PointsHere is a quick example :
Ternary Operator
var name: String = "Michael"
var lastName: String? = "Arango"
lastName != nil ? lastName! : name // Unwraps lastName since is not nil
var name: String = "Michael"
var lastName: String? = nil
lastName != nil ? lastName! : name // returns "name" since "lastName" is nil.
Nil Coalescing Operator.
// This does the same as the ternary operator but in a more elegant way.
var name: String = "Michael"
var lastName: String? = "Arango"
lastName ?? name // Unwraps lastName since is not nil
var name: String = "Michael"
var lastName: String? = nil
lastName ?? name // returns "name" since "lastName" is nil
Good luck