Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial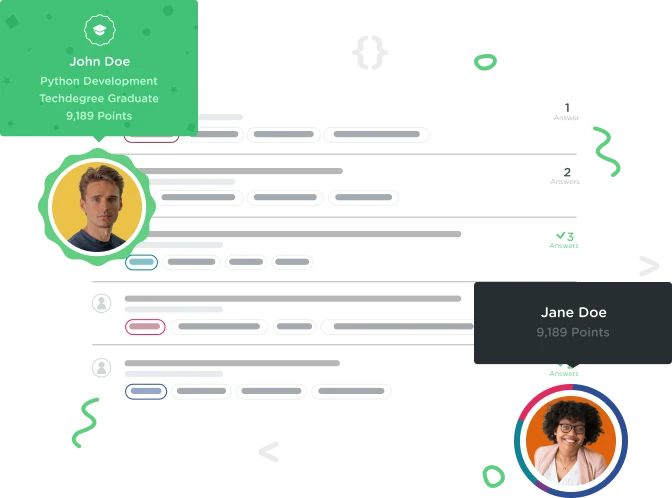
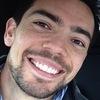
Chris Gaona
Full Stack JavaScript Techdegree Graduate 31,350 PointsA piece of Node.js app works on local machine but not when deployed to Heroku
I've been working on my application and have been deploying it to Heroku after everything that i do to make sure that what I've done still works in production. Everything has been working fine until my recent changes. I've checked to make sure all my dependencies are installed and they are. I have two pieces of code where one works in production and the other does not, but they both work fine on my local machine. Any help would be greatly appreciated.
The following code does work in production:
router.post('/postitem', upload.single('pic'), function(req, res) {
//rename image file to be computer's original file name
fs.renameSync(req.file.destination + req.file.filename, req.file.destination + req.file.originalname);
//Code for posting to json file
var imageText = req.file.originalname;
var titleText = req.body.title;
var newThing = { //new object
"image": "/static/images/" + imageText,
"text": titleText
}
//location of JSON file
if (req.body.select === 'garbage') {
var file = './public/json/garbage.json';
var classItem = "garbage-items";
} else if (req.body.select === 'recycle') {
var file = './public/json/recycling.json';
var classItem = "recycle-items";
} else if (req.body.select === 'greenwaste') {
var file = './public/json/greenwaste.json';
var classItem = "greenwaste-items";
}
//code for search.json
var fileSearch = './public/json/search.json';
var newSearchItem = { //object for search add
"title" : titleText,
"class" : classItem,
"keywords" : ""
}
//Read JSON file for adding search items
var readFile = fs.readFileSync(fileSearch);
//parse JSON file
var parseFile = JSON.parse(readFile);
//push new item to JSON
parseFile['search'].push(newSearchItem);
//stringify file back to JSON format
var stringifyFile = JSON.stringify(parseFile, null, 4);
//Write new JSON to file
fs.writeFileSync(fileSearch, stringifyFile);
//Read main JSON file for adding items
var configFile = fs.readFileSync(file);
//parse JSON file
var config = JSON.parse(configFile);
//push new item to JSON
if (req.body.select === 'garbage') {
config['garbageItems'].push(newThing);
} else if (req.body.select === 'recycle') {
config['recycleItems'].push(newThing);
} else if (req.body.select === 'greenwaste') {
config['greenWasteItems'].push(newThing);
}
//stringify file back to JSON format
var configJSON = JSON.stringify(config, null, 4);
//Write new JSON to file
fs.writeFileSync(file, configJSON, function (err){
if (err) throw err;
req.flash('message', 'Success! Item Added!')
});
//console.logs
console.log('Success!');
//add flash message...should maybe add if successful show this
res.redirect('/dashboard');
});
The following code does not work in production and it is pretty much the same thing. Again, it does work on my local machine though. I'm using npm Multer in this code so I'm not sure if I'm maybe using it wrong or something.
router.post('/postblog', upload.single('image'), function(req, res) {
//rename file to be name from local computer
fs.renameSync(req.file.destination + req.file.filename, req.file.destination + req.file.originalname);
//Code for posting to json file...uses name="" in html form
var imageWords = req.file.originalname;
var titleWords = req.body.name;
var postLink = req.body.name.replace(/\s+/g, '-');
var paragraph = req.body.blogpost;
var author = req.body.author;
var date = req.body.date;
var keywords = req.body.keywords;
var newPost = { //new post object
"title": titleWords,
"link": postLink, //replace spaces with - (dash)
"paragraph": paragraph,
"author": author,
"date": date, //transform to different date format
"picture": "/static/images/" + imageWords,
"keywords": ""
}
//location of JSON file
var postFile = './public/json/blogs.json';
//Read JSON file
var configPost = fs.readFileSync(postFile);
//parse JSON file
var parsePost = JSON.parse(configPost);
//push new item to JSON
parsePost['blogs'].push(newPost);
//stringify file back to JSON format
var stringifyPost = JSON.stringify(parsePost, null, 4);
//Write new JSON to file
fs.writeFileSync(postFile, stringifyPost)
console.log(req.body.name);
console.log(req.body.name.replace(/\s+/g, '-'));
console.log(req.body.author);
console.log(req.body.date);
console.log(req.body.blogpost);
console.log(req.body.keywords);
console.log(req.file);
console.log('Success!');
req.flash('message2', 'Success! Blog Post Added!');
res.redirect('/dashboard');
});
Thank you in advance!!!