Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial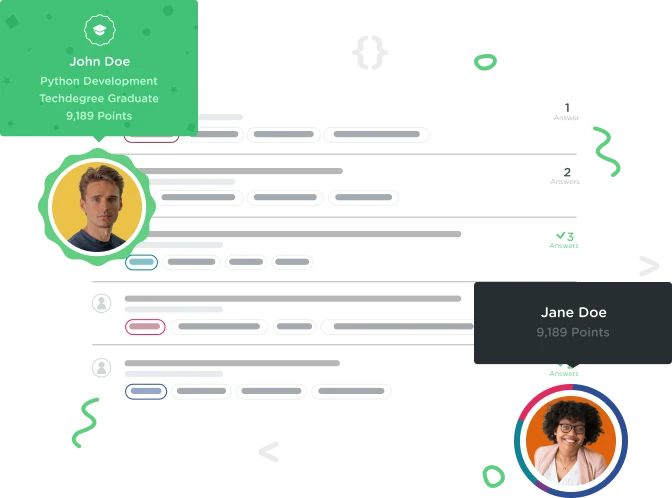

Ben Zuba
13,575 PointsA possible solution for extra code. "multiple students with the same name"
This is my solution to students with the same name. Instead of printing students information when "student.name === search" the student is added to an empty array. Once the loop finishes the newly filled array of matched students is printed to the webpage.
var message = " ";
var student;
var search;
var correct_searches = [];
var found = false;
function print(message){
var output = document.getElementById("output");
output.innerHTML = message;
}
function getStudentReport(student){
var report = "<h2>Student: " + student.name + "</h2>";
report += "<p>Track: " + student.track + "</p>";
report += "<p>Points: " + student.achievements + "</p>";
report += "<p>Achievements: " + student.points + "</p>";
return report;
}
while (true){
search = prompt("Search student records: type a name[Jody] or type 'quit' to close.")
found = false;
if (search === null || search.toLowerCase() === 'quit'){
break;
}
for (var i = 0; i < students.length; i++){
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
correct_searches.push(student);
found = true;
}
}
if (!found){
prompt("That student does not is not in the directory: Press ok to search again")
}
console.log(correct_searches)
for (var i = 0; i < correct_searches.length; i += 1){
student = correct_searches[i];
message += "<h2>Student: " + student.name + "</h2>";
message += "<p>Track: " + student.track + "</p>";
message += "<p>Points: " + student.achievements + "</p>";
message += "<p>Achievements: " + student.points + "</p>";
}
print(message);
}
2 Answers
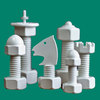
Steven Parker
231,269 PointsSince you've already defined getStudentReport, you could make use of it instead of replicating the code:
for (var i = 0; i < correct_searches.length; i += 1) {
message += getStudentReport(correct_searches[i]);
}
Also, since the loop just accumulates reports in the string "message", you could do that directly in the search loop and eliminate storing the records in an array an processing them later. I think that's more like the original, right? But you could defer the "print" part until the complete string was created.

Carlos Talero Jacome
7,146 PointsGreat work