Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial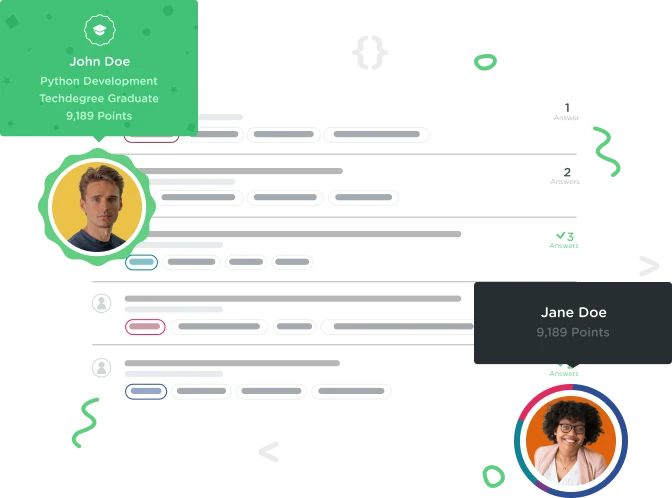
Peter Price
4,007 PointsA problem with my code in task 2 of 2 of the second quiz
Hi,
Does anyone know what is wrong with the code? It just answers "try again"
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public String goKart;
public GoKart(String color) {
mColor = color;
GoKart goKart = new GoKart ("red");
mBarsCount = 0;
}
public boolean isBatteryEmpty(){
return mBarsCount == 0;
}
public boolean isFullyCharged(){
return mBarsCount == MAX_BARS;
}
public String getColor() {
return mColor;
}
public void charge() {
}
}
4 Answers
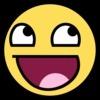
Michael Hess
24,512 PointsHi Peter,
It looks like there is a GoKart object declared in the constructor.
It may help to see the completed challenge. This is a full solution to both tasks:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public boolean isBatteryEmpty(){
if(mBarsCount == MAX_BARS){
return false;
}
return true;
}
public boolean isFullyCharged(){
return mBarsCount == MAX_BARS;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_BARS;
}
}
Hope this helps! If you have any further questions feel free to ask!
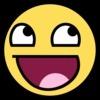
Michael Hess
24,512 PointsHi Peter,
Everything looks okay, but the isBatteryEmpty() method should be modified.
Try this:
public boolean isBatteryEmpty(){
if(mBarsCount == MAX_BARS){
return false;
}
return true;
}
If you have any other questions feel free to ask! Hope this helps!
Peter Price
4,007 PointsHi Michael,
Thanks so much for your reply. I tried your code in mine, like so:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public String goKart;
public GoKart(String color) {
mColor = color;
GoKart goKart = new GoKart ("red");
mBarsCount = 0;
}
public boolean isBatteryEmpty(){
if(mBarsCount == MAX_BARS){
return false;
}
return true;
}
public boolean isFullyCharged(){
return mBarsCount == MAX_BARS;
}
public String getColor() {
return mColor;
}
}
But it says:
JavaTester.java:89: error: cannot find symbol
kart.charge();
^
symbol: method charge()
location: variable kart of type GoKart
JavaTester.java:123: error: cannot find symbol
kart.charge();
^
symbol: method charge()
location: variable kart of type GoKart
2 errors
I will try and find an answer, but I would appreciate it if you could have a look and try and find the problem.
Peter Price
4,007 PointsAnother thought,
Do I need the isFullyCharged and getColor methods (which are at the bottom of the first code block).
Peter Price
4,007 PointsThanks Michael!! That was a great help.
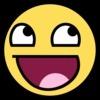
Michael Hess
24,512 PointsMy pleasure!
Peter Price
4,007 PointsPeter Price
4,007 PointsThis is the Java Objects, Helper Methods quiz question: "Now create a helper method named isFullyCharged. It should return true if the GoKart is at max capacity. "