Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial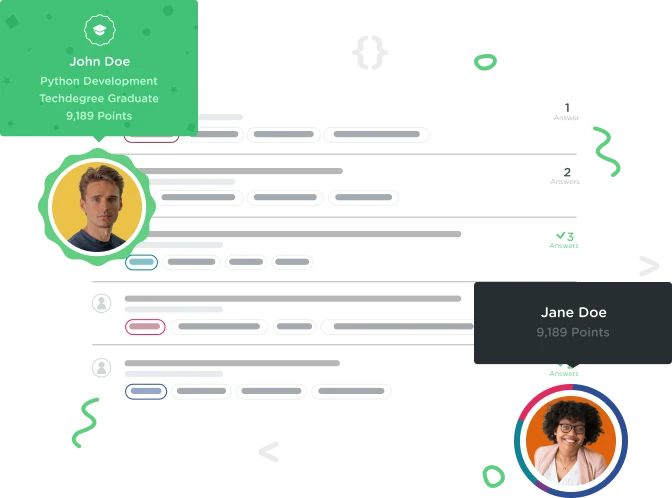

Adam Beck
9,345 PointsA question about 'class'
So i've tried a wide range of answers with no luck. I left my answer 'as is' and wanted to see if anyone knew what I am doing wrong. Having trouble with "self.location" and assigning it with 'struct Location'. Here is the question..
"In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values.
For this task we want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location.
In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness."
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init (name: String, location: Location){
self.name = name
self.location = Location(latitude: Double, longitude: Double)
}
}
let someBusiness = Business(name: location: )
2 Answers

Michael Reining
10,101 PointsHi Adam,
You are very close. The initializer for the Business is wrong. If a class contains a Type of another class, you only need to link it to the type. The initialization only happens when you create an instance of the class.
See details below.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init (name: String, location: Location) {
self.name = name
self.location = location // just link it to parameter name above
}
}
let someBusiness = Business(name: "Some business",
location: Location(latitude: 45.5,longitude: 50.0)) // initialize location
I hope that helps,
Mike
PS: If you found the above helpful, be sure to check out my app which breaks code challenges down and really explains things so you can learn faster.
Code! Learn how to program with Swift
https://itunes.apple.com/app/code!-learn-how-to-program/id1032546737?mt=8
PPS: I could not have developed the above app without going through the awesome resources on Team Treehouse. Stick with it. It is so worth it!
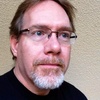
Chris Freeman
Treehouse Moderator 68,425 PointsThere are two errors to fix.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location){
self.name = name
self.location = location // assign value passed to parameter
}
}
// let someBusiness = Business(name: location: )
// Provide all attributes to the initializer. Use mock data
let someBusiness = Business(name: "Test", location: Location(latitude: 10, longitude: 20))
Adam Beck
9,345 PointsAdam Beck
9,345 PointsThank you! I will definitely check that out. The Treehouse community has been nothing less than awesome!