Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial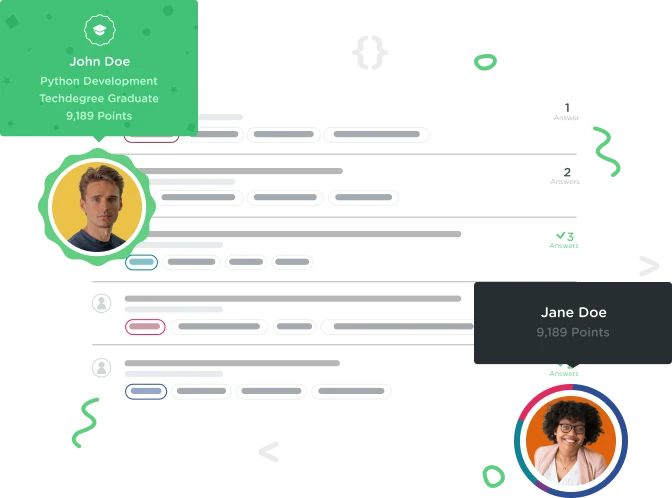

Anthony Lopez
963 PointsA question about iterating...
How does this work exactly?
for book in books:
Does it know to iterate each item in the list because you removed one letter from the variable, or does it understand that book is the singular form of books?
As an example:
tacos = [ "Lengua", "Al Pastor", "Carne Asada", ]
for taco in tacos:
This would iterate between each item in the list.
But if instead I used:
taco = [ "Lengua", "Al Pastor", "Carne Asada", ]
Would I type out
for tac in taco:
Or would I need to a word in the plural form if I'm making a list?
5 Answers
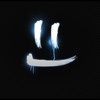
Grigorij Schleifer
10,365 PointsHey Anthony, this is an important question!
The general form of a for loop is:
for some_meaningful_word_you_choose in list_name:
# assigned values from object_name to some_meaningful_word_you_choose
# will be printed to the screen one after another
print(some_meaningful_word_you_choose)
The for loop "knows" how many items there are in a list and assigns every one item from that list to the some_meaningful_word_you_choose. Ideally, you pick a word that is meaningfull to your and your team. Often you choose a character i or key for that purpose. But you can pick whatever works well for you.
This would work:
for i in tacos:
# every member of tacos will be assigned to i and printed to the screen
print(i)
Remember to pick variable names and names in the loops that explain the purpose, so the future YOU understands the code.
Does this answer your question?
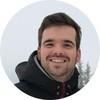
John Lack-Wilson
8,181 PointsHey Anthony, I understand the confusion here, but there's no way for python (or really any language) to understand that by removing a letter it is a singular version. The only rules are that the first variable cannot be the same name as the second (+ not a keyword, builtin, etc). You are simply naming a variable to use within the loop, and each time it goes back to the top of the loop that variable is re-assigned a new value from the list (or whatever you are iterating over)
Examples:
cars = ["BMW", "Audi", "Mercedes"]
for german_car_manufacturers in cars:
print(german_car_manufacturers)
for television_programmes in cars:
print(television_programmes)
for c in cars:
print(c)
for car in cars:
print(car)
Generally it would be sensible to name the first variable the singular version of the second so that both you and anyone reading your code can understand the loop. The examples I gave were all pretty silly except for - for car in cars, as this is obvious what 'car' is referring to.

Anthony Lopez
963 PointsThis is a great answer, thank you John!
It makes sense to me now.
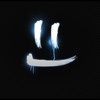
Grigorij Schleifer
10,365 PointsHi Walter, I am not sure I understand your question. Every item is assigned to i one at a time. So every loop you will have another item that is "represented" by i. You can access that item inside the for loop or inside the function that the for loop belongs to. Or you assign i to another variable (a list for example) and use it somewhere else. Does it make sense?

Truman Moore
11,964 PointsThanks guys, I also did not realize how python understood that continent was the singular form of continents.

walter olazabal
7,407 PointsHi Grigorij, what I meant when you create the loop you are creating a variable of each item on that list right? so my question was after the loop what is inside the variable i is it empty or does it still contain the items of the list ?
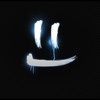
Grigorij Schleifer
10,365 PointsThis is a very nice question. The short answer is YES. Run this code chunk in your editor and you will see that the last assigned item is stored outside of the loop scope and you can use it.
tacos = ["Lengua", "Al Pastor", "Carne Asada", ]
for index, taco in enumerate(tacos):
print(str(index), ":", str(taco))
print("The last item from the list is: ", str(taco))
The output is:
0 : Lengua
1 : Al Pastor
2 : Carne Asada
The last item from the list is: Carne Asada
[Finished in 0.133s]
Check out this stack overflow discussion.
I hope this is helpful
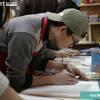
Cao Tru
Courses Plus Student 1,103 PointsHi Grigorij Schleifer can you explain for me why index variable can output is number (ie: 0,1,2,..) what happen if i add more variables in for loop:
tacos = ["Lengua", "Al Pastor", "Carne Asada", ]
for a, b, c, index, taco in enumerate(tacos):
print(str(index), ":", str(taco))
print("The last item from the list is: ", str(taco))
```
Anthony Lopez
963 PointsAnthony Lopez
963 PointsPerfect thank you! This makes a lot of sense. It was unclear to me whether or not the i portion in the for loop was arbitrary or not.
walter olazabal
7,407 Pointswalter olazabal
7,407 PointsI have another question, so the items inside the list are assigned the name "i", is this only inside the for loop? or are the items inside the list now assigned the "i" overall?
Luke Maschoff
820 PointsLuke Maschoff
820 PointsWow, thanks a lot! So if I could sum things up here for myself, the for in statement takes each value of the list, and assigns it to a variable? For example: