Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial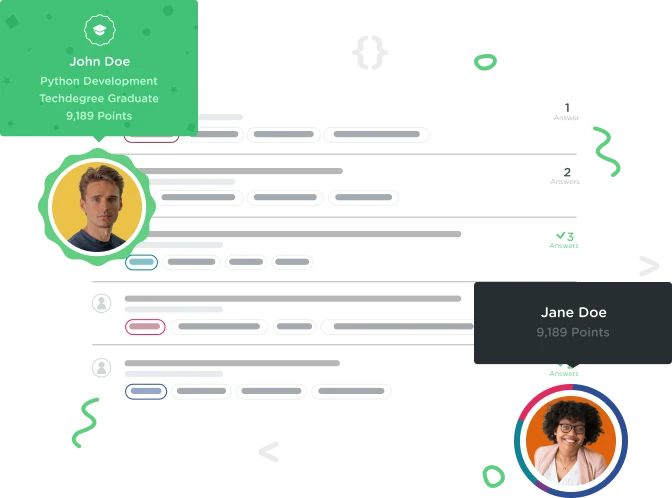

Cuong Hoang
1,769 PointsA question about prompt(); ...
So whatever the user or we input in prompt(); , it will always return the input or value as a string?
2 Answers
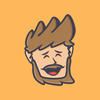
Sam Baines
4,315 PointsYes correct - but you can easily convert the string from a prompt command into an integer providing the string starts with a number, using the code below:
var width = prompt('Enter the number 190px');
parseInt(width); //This will convert the string "190px" from the width variable into the integer 190.
And of course you could just use the function parseInt straight onto the prompt() function too:
var width = parseInt(prompt('Enter a number'));
Hope this helps.

Cuong Hoang
1,769 PointsThanks for the replies guy, but as a beginner it's really hard to understand the base 10 system.. do you mind explaining?
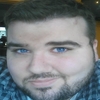
Marcus Parsons
15,719 PointsThe base 10 system is the standard number system from 0-9 (10 numbers). It is the standard system in use with mathematics.
But there are also other number systems such as Octal (8 number system) or Hexadecimal (16 number system). Octal isn't used that much, but Hexadecimal is very frequently used to express colors as it has a fast, efficient way of representing blocks of numbers that can convert into the RGB color scale.
In JavaScript, as of ECMAScript 5, in order to get an octal number, the radix must be explicitly set when parsing integers. They can't be parsed from a string representation.
//the first argument is the string to parse
//the second argument is known as the radix
//which sets which number system to use
var anOctal = parseInt("17", 8);
//Puts 15 into console
console.log(anOctal);
I don't want to confuse you too much with all of this. If you want to hear more, though, I will gladly go on.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsYou always want to explicitly set that you are parsing the number using the base 10 system, however. In certain cases, certain strings will register as hexadecimal numbers unless explicitly set otherwise. Here is how you should write parseInt (99% of the time unless you're using a different system of numbers):
var width = parseInt(prompt('Enter a width:'), 10);
Sam Baines
4,315 PointsSam Baines
4,315 PointsThanks thats good to know marcus - I was just going by what I learnt in the javascript basics course already - I will need to do some looking into this base 10 system.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 Pointshttps://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt
Read under the heading Description which lists possible ways that parseInt can get the wrong number. Here's something to try in your console:
Sam Baines
4,315 PointsSam Baines
4,315 PointsAh glad you have made me read this Marcus, I fully understand it now - so the base 10 system is the main one to use for just generic numbers based around 0-9 and base 16 if you were trying to parse integer values for example for a RGB value from hexadecimal color codes.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAbsolutely! You could break apart a hexadecimal color value gathered from CSS (or something) and convert it into rgb:
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsOr:
Sam Baines
4,315 PointsSam Baines
4,315 PointsAwesome Marcus - thats a great little tutorial code right there for converting hexadecimal codes into rgb (glad I was right about using the base 16 for this, otherwise I would have look like a right newbie) - I'm off to have a play with this!
ps. you also just taught me how to use the the .replace method.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAwesome man! Replace is a very powerful command and once you get the hang of regular expressions, it can be used to do some really cool things. Play around with it and see what you come up with :)