Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial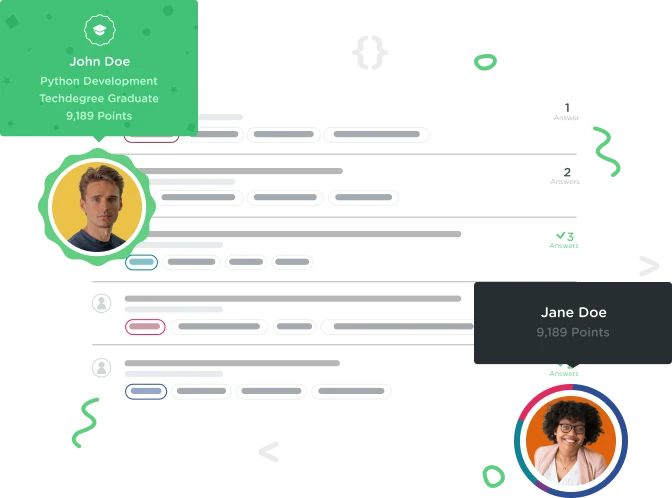

Julian Ptak
30,920 PointsA question about syntax and semantics... PHP Object Oriented Programming Final Challenge
Spoilers peeps so if you haven't beaten the challenge, please don't read any further. The answer is below:
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish
{
public $species;
function __construct($name, $flavor, $record, $species)
{
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
public function getInfo()
{
return $this->species . " " . $this->common_name . " tastes " . $this->flavor . ". The record " . $this->species . " " . $this->common_name . " weighed " . $this->record_weight . ".";
}
}
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook");
echo $brook_trout->getInfo();
?>
Trust me there is definitely a question...
Now this code works, it passes the challenge and what-have-you. But my question here is about the ongoing use of "$this". Clearly it works but I've been told when programming object-oriented stuff in Java that using "this" too frequently can lead to code that is more difficult to understand. Often when programming larger projects using multiple objects and inheritance, I've noticed (in Java) that I've gotten some strange errors due to variable pointers pointing to the wrong object because of an incorrect use of "this". Bear with me now... here's the question:
Is there a way in PHP to do the return output from getInfo without using "$this"?
I'm talking about something that looks more like this:
//... other code up here
public function getInfo()
{
return $species . " " . $common_name . " tastes " . $flavor . ". The record " . $species . " " . $common_name . " weighed " . $record_weight . ".";
}
Sure, the problems I had in Java were probably largely due to my own inexperience but by passing stuff to variables or not using globals so much, I was able to avoid a lot of issues... in Java. So this question is more of a "best practice" question... does anybody have any suggestions regarding object oriented variable referencing in PHP? Is the former code the best way to handle an object's variables in PHP or should global variables be avoided or at the very least referenced without using "$this"?
Thanks for your time! Looking forward to hearing your ideas!
2 Answers

Jesse Richard
1,899 PointsFrom Wiki:
"Object-oriented programming (OOP) is a programming paradigm that represents concepts as objects that have data fields (attributes that describe the object) and associated procedures known as methods."
This is effectively saying in the first line of the description of OOP that you should be referencing the class variables and objects rather than passing params to a method or a function (when it can be helped).
I can think of a few scenarios in which passing variables to a method, specifically an outside method, would be the long way of getting to the final product.
$this is better abstracting. $this is better practice.

thomas howard
17,572 PointsGreat question and enjoyed the answer.