Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial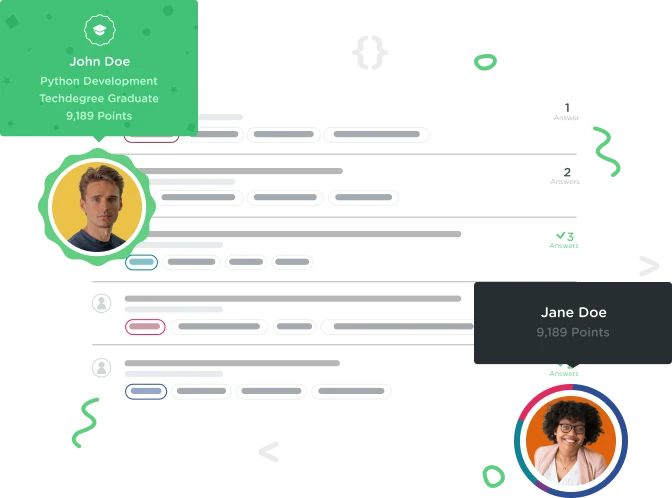

pawkan kenluecha
26,303 PointsA question about the "infinite loop".
def get_guess(bad_guesses, good_guesses):
while True:
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
elif not guess.isalpha():
print("You can only guess letters!")
else:
return guess
This is infinite loop code block from the lesson. As per my understanding, there are two options to operate with this kind of loop. The first one is "break", this will break out of the loop. The second is "continue", this will skip the command after its line and continue the next round of the loop.
I want to recheck this because the code above it doesn't have any those command. So I incline that the "return" statement should return "false" to while loop somehow. But, I don't know the real mechanics over this. Could someone verify my statement above and give me more information on the mechanics of "return"?
1 Answer
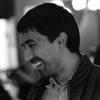
Martin Cornejo Saavedra
18,132 PointsThe script you wrote is a function
def get_guess(bad_guesses, good_guesses):
So, the return statement is returning that function's value, ending the loop. For example:
letter = get_guess(['a', 'b'], ['c', 'd'])
print(letter)