Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial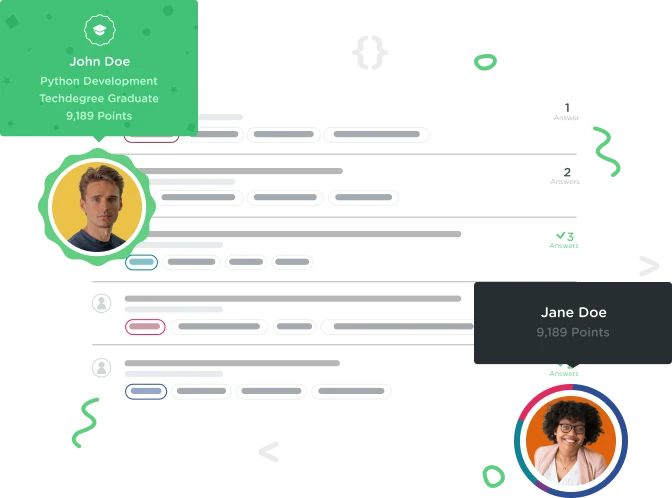

taejooncho
Courses Plus Student 3,923 PointsA question in combo task. ( I solved it, but I want to know why my first attempt did not work)
You can see my question in the code as comments, but to summarize:
- why did the first attempt code not loop until num was bigger than the length of values in x or y?
- What is the difference that made second block code to work but not work for the first block of code
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# This was my first attempt to answer this question
def combo(x, y):
combo_list = []
num = 0
for values in x , y:
results = (x[num], y[num])
combo_list.append(results)
num += 1
return combo_list
# When I tired to run my first attempt code on eclispe
# comb([1, 2, 3, 4, 5], "abcde")
# I am not sure why, but for some reason, the output is
# [(1, 'a'), (2, 'b')]
# combo_list was not appending the remaining value in x or y.
# since I included num += 1 at the end of for loop
# I thought that all the values in x and y will be included
# until the index num value is bigger than total values in x or y
# After struggling for an hour I solved this task with this code
def combo(x, y):
combo_list = []
num = 0
for values in x: # I took out y
results = (x[num], y[num])
combo_list.append(results)
num += 1
return combo_list
# when I ran this, the combo_list included all the values
# unlike the first attempt, it included all the values of x and y
# Consequently, my question is
# 1. why did the first attemp code not loop until
# num was bigger than len of values in x or y?
# 2. What is the difference that made second block code to work
# but not for the first block of code
1 Answer

Jon Mirow
9,864 PointsHi there!
It's happening because you're creating a tuple of only two elements, so python goes through to the first element in the tuple (x) and gets the list [1,2,3,4...] and that becomes value. Then the next loop it goes through the second element in the tuple and value becomes the string "abcd..." After that the tuple is over and the for loop ends.
To demonstrate:
>>>for name in "Bob", "Jane":
print(name)
'Bob'
'Jane'
Note how it doesn't print 'B' - 'o' - 'b' - 'J' - 'a' - 'n' - 'e' ?
It works when you remove y, because now it's not a multidimensional tuple anymore, but a flat list you're iterating through and so the for loop goes through each item in the list x. Because y and x are always the same length in this example, it works fine whichever you loop through - you could do
for i in range(len(x)):
And use i rather than keeping track of num :) In the real world, you'd probably want to do some check that both inputs are the same length and only loop the length of the shorter if not to avoid an IndexError, but that's not needed here.
Hope it helps :)
taejooncho
Courses Plus Student 3,923 Pointstaejooncho
Courses Plus Student 3,923 PointsThank you! Jon for your help last time and this time!!
Your explanation is really good! Even though my question was worded very confusingly. Now I understand why the results were coming like that! I will also take note of your suggestion!