Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial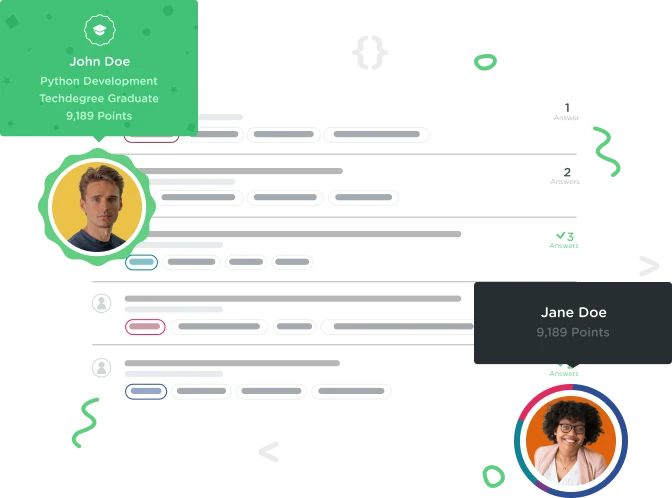

John Smith
2,573 Pointsa question on pointers
In this video http://teamtreehouse.com/library/objectivec-basics/beyond-the-basics/pointers-part-2
at 4:02, line 28, I don't understand why the program printed out a different memory address.
First, you pass &i (the memory address of i) as the argument to the function. line 28, printf("The memory address of x = %p\n",&x);
What exactly is &x? Is it the memory address of where the pointer &i is stored at?
As I understand it,
the data for i is stored in memory, &i is a pointer that points to the location of this memory, put this pointer must also require memory to be alive (the compiler automatically keeps track of this),
So when the function is printing out &x initially, it's printing out the memory address of the pointer and not where the pointer is pointing to.
Am I correct with this? Please help because this is very confusing.
10 Answers
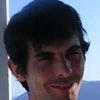
Jeremy Smith
1,395 PointsThe reason being it won't ever be the same is because it's essentially 'Random' Access Memory, Access being in single quotes as it's the operative word (or rather Random being the operative word here).
Even if you were to keep running the same application multiple times each time there'd be a different address to where that memory is stored.
It's quite essentially any memory allocation you create (either in main or otherwise (in other functions even)) will always be set in runtime, so on each run that means it'll change.

John Smith
2,573 PointsI just don't understand what &x is referring to. In the final code, we print out x, which is the pointer pointing to the data of int i. But at 4:02, we said &x. It's almost like saying a pointer of a pointer.

John Smith
2,573 PointsAnother question I have in objective c, are object pointers or the actual data itself.
For example,
NSNumber *number = [[NSNumber alloc] init]; number = .....
In the second line, are we manipulating the data itself through the pointer?
Why do we not say *number = ....
What if we were to print out the memory address of number and *number? Would they be different at run time? Meaning, they refer to different memory location, one points to the data and one to the location where the compiler manage the pointer.
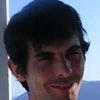
Jeremy Smith
1,395 PointsIn a nutshell anything that has the & sign in front of it in C is a memory address.
So if you had say: 'int myvar = 5;' // very quick and dirty example
To make a pointer variable that saves the memory 'address' of myvar you'd do:
int foopointer = &myvar;
So now foopointer has only the memory address of myvar.
If you were to have &myvar in printf() it'd output the memory address of myvar.
To answer your second question every time you go to run something that illustrates the above the memory address of myvar would change each time you run it.
The asterix * is to dereference a pointer, I was told by a person I used to work with, it's when you're happy with the data that the pointer holds (it's valid) then you only dereference. You can have pointers (because of them being declared on what's called the heap, as opposed to stack when you do stack programming, like assembly) you can find memory allocations can get corrupted.
This is what you strongly want to avoid at all costs.

John Smith
2,573 PointsWhat is you were to print &foopointer? It would be a separate location than &myvar right?
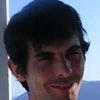
Jeremy Smith
1,395 PointsThat holds the memory allocation of myvar, but yes you're correct with that one.
They do take some getting used to and getting your head around but once you learn the basics it's pretty simple to be honest.

John Smith
2,573 PointsOk, thanks. But I don't understand why when you're manipulating objects in objective-C, the syntax doesn't include *.
Initially when you're allocating an object, you specify that is is a pointer.
NSNumber *number = [[NSNumber alloc] init];
However, if you want to assign it something, you can just say, number = @23
This syntax makes it seem like you're manipulating the memory of the pointer instead of the data that the pointer is pointing to. Something like this would make more sense,
*number=@23 Of course, if you try to do this, you'll get a syntax error?
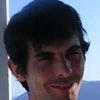
Jeremy Smith
1,395 PointsActually just run a very basic example to prove this t o myself and the value of the points not changing.
But naturally it'd be different from what the instructor has, because I mean every single thing that your computer loads would be needed to be loaded into memory, even the processor has drivers as far as the Operating System's concerned (though naturally it'd be further up the memory allocations at like the first one or something, for speed of access, even the graphics cards drivers be it a simple bog standard graphics card or a really fully 3d with physix or whatever you call it from nvidia (be a longer range of memory address)).
I wouldn't worry too much for now, as long as it's outputting a value of an integer you're good to go.
Also to note on the Bloodshed Dev-C++ compiler, says (when I compile what you were asking) '[Warning]: initialization makes integer from a pointer without a cast.'

John Smith
2,573 PointsI'm not confused on the fact that it's different, but on whether or not we are dealing with two separate memory location at the same time.
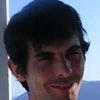
Jeremy Smith
1,395 PointsYou would be yes, since they on a technical level store different sets of data.