Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial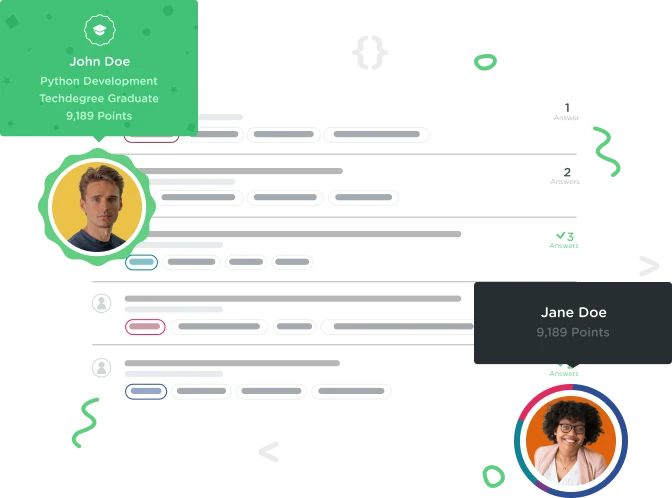

Nikolaj Bewer
1,150 PointsA question regarding my list(words) from which random.choice picks
I thought I would modify my hangman game just a little. I want to build hangman game that covers all the EU capitals. Unfortunately the capital from 'san marino' is 'san marino' and consists of two words. Now, if random.choice(words) picks 'san marino' I would like the game to add a space without an underscore between those words. Below I will add my current code. I know that the way it is right now it won't work. I have tried strings with split and lists, but none of these ways worked out for me. Can anyone help me?
import os
import random
import sys
words = 'sanmarino berlin '.split()
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def draw(bad_guesses, good_guesses, secret_word):
clear()
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
for letter in bad_guesses:
print(letter, end=' ')
print('\n\n')
for letter in secret_word:
if letter in good_guesses and letter in secret_word[0]:
print (letter.upper(), end='')
elif letter in good_guesses:
print (letter, end='')
else:
print('_', end='')
print('')
def get_guess(bad_guesses, good_guesses):
while True:
guess = input("Guess a letter: ").lower()
if guess=='exit':
clear()
print("Bye!")
sys.exit()
elif len(guess) != 1:
print("Only guess one letter at a time!")
elif guess in bad_guesses or guess in good_guesses:
print("You've guessed that already")
elif not guess.isalpha():
print("Only letters")
else:
return guess
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in secret_word:
if letter not in good_guesses:
found = False
if found:
clear()
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
print("You win!")
print('')
print("The secret word was {}".format(secret_word))
done = True
else:
bad_guesses.append(guess)
if len (bad_guesses) == 7:
draw(bad_guesses, good_guesses, secret_word)
print("You lost!")
print("The secret word was {}.".format(secret_word))
done = True
if done:
print('')
play_again = input("Play again? y/n ").lower()
if play_again != 'n':
return play(done=False)
else:
sys.exit()
def welcome():
start = input("Press enter/ return to start or Q to quit ").lower()
if start == 'q':
print("Bye!")
sys.exit()
else:
return True
print('Welcome to Capital Guess!')
done = False
while True:
clear()
welcome()
play(done)
1 Answer
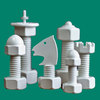
Steven Parker
231,269 Points
You got pretty close.
I can think of two changes needed to the original game to accommodate spaces:
- ignore spaces when checking for a win
- explicitly print spaces when showing the current status
For the win checking, you could do this:
for letter in secret_word:
if letter not in good_guesses and letter != ' ': # ignore spaces
found = False
And when printing the current status:
for letter in secret_word:
if letter == ' ': # always print spaces as-is
print(' ', end='')
elif letter in good_guesses:
print (letter, end='')
else:
print('_', end='')
Also, put the word list back to an ordinary array:
words = ['san marino', 'berlin']
Now that spaces are allowed, you could also guess at entire phrases, such as these examples from the "Wheel of Fortune" game:
words = [
'pizza party', # event
'a long shot in the dark', # before and after
'ants in your pants', # rhyme time
'dog and buttermilk biscuits' # same name
]
Nikolaj Bewer
1,150 PointsNikolaj Bewer
1,150 PointsHi Steven, Thanks a lot for your answer this really helped me out a lot. Now I came up with the idea to combine hangman with a quiz. The code is the following one:
... the rest of the code is up in my post.
So I want random.choice(words) to pick a word (capital) from my list. And then post the appropriate state to it as a question.
Now this code works perfectly fine and I could extend the code by all the " elif secret_word in words[1]: print('What is the capital of the {}?'.format(states[1]))" statements and always increase the number by one but that would be quite exhausting.
So I tried something like this:
But unfortunately this doesn't work out at all.
Do you have any idea how to loop through all the states in a short and elegant way?